Have you ever gone through the code written by someone else, having meaningless identifiers and code? If yes, you must know how frustrating it is to understand and work through such code. During development, most developers ignore the fact that someone else might maintain their code. They write code that they can understand. However, it becomes difficult for them to understand it after some time.
Magic numbers are numeric values used in code that may be difficult to identify or remember the purpose of. Magic numbers are often used to represent things like status codes, message types, or error codes. Magic numbers are a bad practice since they make code harder to read and understand.
In this article, you will learn about magic numbers and find out how magic numbers can hurt your code or not.
What are magic numbers?
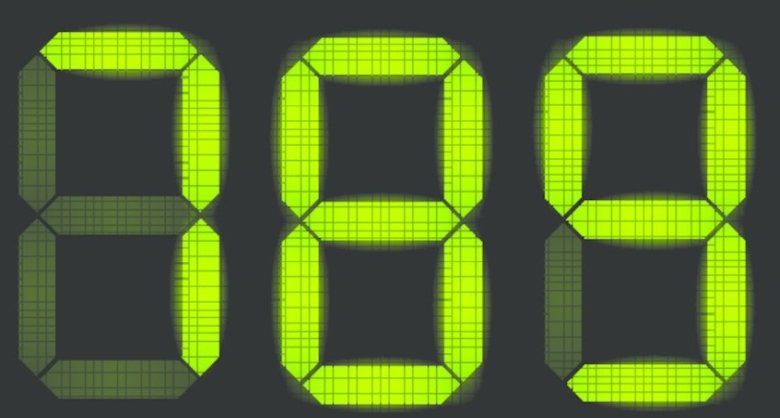
Magic numbers refer to the numbers used directly in the code rather than storing them in some meaningful variable. Using magic numbers is considered a bad practice in programming as they can cause ambiguity and confusion.
They also make your code useless for other developers as they cannot understand what your code does and how.
Besides the general definition of magic numbers, you can also call the following objects a magic number:
- Undefined constant values are used twice or more in the source code.
- Meaninglessly defined variable names that do not express their purpose.
- Unique values used in certain parts of the code without any global meaning.
Here is a simple example of magic numbers.
//magic variable
int x = 5;
//magic variable
int y = 4;
//magic variable
int z = x - y;
Console.WriteLine(x + "-" + y + "=" + z);
In the above code, x, y, and z are magic variables as they do not define their purpose or they do not represent any meaning. However, to make them meaningful, you can define ‘x’ as number1, ‘y’ as number2, and ‘z’ as difference, as shown in the following code.
//Better approach
int number1 = 5;
int number2 = 4;
int difference = number1 - number2;
Console.WriteLine(number1 + " - " + number2 + " = " + difference);
Similarly, the code below has a magic number. It’s hard to know what the 6 represent.
if (numberOfOrderedItems > 6) //Here 6 is the magic number
{
//some processing logic
}
The solution for magic numbers is to define and use a symbolic constant instead.
int MINIMAL_ORDER_ITEMS_TO_APPLY_DISCOUNT = 6;
if (numberOfOrderedItems > MINIMAL_ORDER_ITEMS_TO_APPLY_DISCOUNT)
{
//some processing logic
}
Now it’s easier to understand that 6 represents the threshold for whether to apply to discount to the order or not.
Magic numbers have their siblings in magic strings, which you can read all about in a separate article.
Now that you know what magic numbers are, let’s see the problems they cause.
Why are magic numbers bad?
Using any magic numbers is a bad practice in programming. There are various reasons behind it, some of which are as follows:
- Magic numbers destroy the simplicity and maintainability of your code.
- They are ambiguous, meaning they do not define their purpose or do not have one. They can easily confuse the new as well as the experienced programmers.
- Magic numbers with multiple occurrences make it difficult to modify the code as the developer has to look for them throughout the code and change them one by one. This way, they cause a waste of time and energy.
- With magic numbers, the chances of errors increase. Especially with big numbers, it becomes difficult to trace the wrong digit and change it.
By using the magic numbers, you can make your code more and more error-prone and difficult to maintain and understand.
However, not using the magic numbers bring many benefits:
- Improved code completion provided by the IDEs and text editors.
- You get more understandable, maintainable, modifiable, and reusable code.
- You can have meaningful documentation.
How to eliminate magic numbers in C#?
After knowing all the downfalls of magic numbers, you might wonder whether it is possible to avoid them or not.
Good news!
Eliminating them from your C# code is not a big deal, especially if you are working with Visual Studio. You can easily avoid magic numbers using the appropriate identifiers and other approaches. However, if you already have written a lot of code with many magic numbers, do not worry.
Refactoring methods in Visual Studio greatly aid programmers as they automatically suggest the best possible solution to your meaningless code. Visual Studio has the “Introduce constants” refactoring to eliminate magic numbers, which autonomously suggests creating the constants with meaningful names and using them throughout the code.
Introduce constant refactoring in Visual Studio
Here is how you can use introduce constants refactoring:
- Step 1: Go to the magic number and click on it.
- Step 2: Click on the lightbulb or screwdriver in the gutter or press CTRL + .
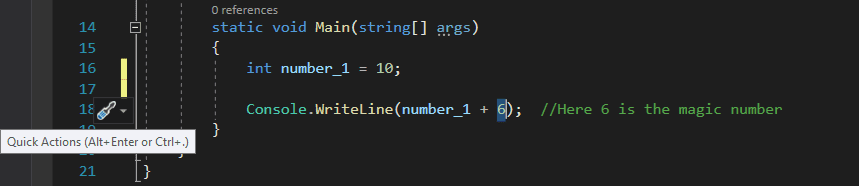
- Step 3: The first option you see will suggest you to introduce the constant while explaining how it will change your code.
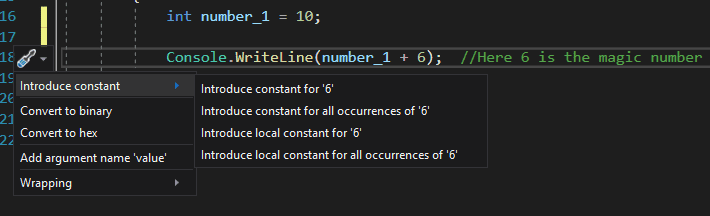
- Step 4: Choose any of the four options shown in the following image as per your preference:
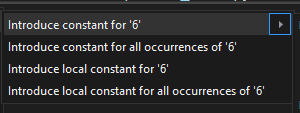
- Introduce constant for 6 will create a constant for the current occurrence of the magic number.
- Introduce constant for all occurrences will declare a constant for global usage.
- Introduce local constant will initialize the constant in the current scope.
- Introduce local constant for all occurrences will define a local constant in all the scopes where the magic number is used.
- Step 5: Choose the name of the constant as per your choice.
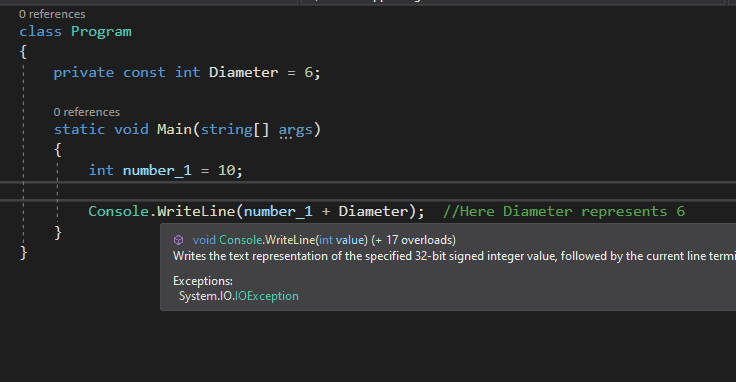
This way, you can refactor all the magic numbers in your C# code.
Conclusion
Magic numbers can cause you and other programmers much trouble due to their anonymity. They make your code more vulnerable to errors and reduce the maintainability and reusability of your code.
The good programming principle is to avoid magic numbers as much as possible and use named constants and values to avoid confusion.