The ordering of parameters of a function may seem less important than other program concerns.
Thus, you might randomly order them in most of your functions.
Nonetheless, this random ordering can impact a program’s consistency, clearness, and readability. Therefore, let’s learn in this article the key best practices you must follow when ordering the parameters of a function.
What are the best practices for ordering parameters in a function?
The best ways you can arrange the parameters of the function are using your common sense and considering the importance, required and optional parameters, and the ability to group the parameters together.
1) The common sense
Use common sense and order the parameters to feel most natural for the function caller.
For example, most programming languages contain functions to copy a file from a source to a destination. The function signature has the source parameter first and the destination parameter second. Passing the source parameter as the first argument sounds more natural to the function caller than specifying it last. As a result, it is self-descriptive and clearly conveys the purpose and behavior of the function to the caller.
Let’s take another example and understand it more clearly. Suppose your program has a function that adds x and y coordinates into a list. Then it is more natural to specify the x coordinate first and the y coordinate second and do the processing in that order.
static void AddCoordinates(int x, int y);
Specifying y coordinate first is obviously not natural.
static void AddCoordinates(int y, int x);
2) The importance
If there is no such direct natural ordering of parameters, consider their importance.
The best practice is to define the most important parameter first.
For example, suppose your program needs to add employee data to a database. In a database, the employee ID parameter is essential to create a unique record for each employee. Therefore, it is important to first declare the employee ID parameter in the function call. The next parameters can be ordered based on how important those parameters are for your application.
The following code explains this.
The program gets the employee ID, first name, and last name from user input. Then it calls the InsertEmployee
function to insert those data into the DB. In this case, the function signature should be like the one below.
namespace Example;
//Declare Employee class
public class Employee
{
public int ID { get; internal set; }
public string FirstName { get; internal set; }
public string LastName { get; internal set; }
}
class Program
{
public static List<Employee> employees = new List<Employee>();
static void InsertEmployee(int employeeId, string firstName, string lastName)
{
Employee emp = new Employee
{
ID = employeeId,
FirstName = firstName,
LastName = lastName
};
employees.Add(emp);
Console.WriteLine($"Adding Employee ID: {emp.ID}, " +
$"Name: {emp.FirstName} {emp.LastName}");
//save to DB
}
public static void Main(String[] args)
{
Console.WriteLine("Enter employee ID: ");
int employeeId = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter first name: ");
string firstName = Console.ReadLine();
Console.WriteLine("Enter last name: ");
string lastName = Console.ReadLine();
//call the method
InsertEmployee(employeeId, firstName, lastName);
}
}
3) Required and optional parameter ordering
The third important thing you need to consider is your function’s required and optional parameters.
In C#, required parameters are mandatory and optional ones are parameters you can omit. All required parameters need to come before optional parameters. If you do not specify the value for the optional parameters, default values will be attached.
You may want to define functions with a mix of required and optional parameters, especially in designing API endpoints.
For example, a function that returns weather information needs two mandatory parameters; the location’s latitude and the longitude. The optional parameter would be the ‘unit system’ to specify the unit of the weather measurement.
Consider the following C# code example. The SendEmail
method takes five parameters: three are required, and two are optional. The required parameters are listed first, followed by the optional parameters.
class Program
{
static void SendEmail(string recipientEmail,
string subject,
string body,
string cc = "manager@gmail.com",
string bcc = "group@example.com")
{
Console.WriteLine("Sending email to: " + recipientEmail);
Console.WriteLine("Subject: " + subject);
Console.WriteLine("CC: " + cc);
Console.WriteLine("BCC: " + bcc);
Console.WriteLine("Body: " + body);
}
static void Main(string[] args)
{
// Required parameters
string recipientEmail = "tess123@gmail.com";
string subject = "Request for a leave";
string body = "Example email body";
SendEmail(recipientEmail, subject, body);
}
}
This produces the following output:
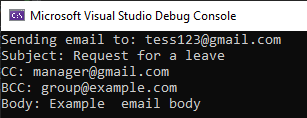
4) Group-related parameters
When ordering parameters, check if there are related parameters that you can specify together.
For example, let’s say your program has a function that draws a particular shape. The arguments related to the shapes’ size, color, and thickness can be specified together.
This parameter grouping helps programmers to define similar parameters quickly and improves self-descriptiveness.
The following C# program explains how you can group similar parameters.
The parameters ‘width’ and ‘height’ related to the rectangle size have been defined first, followed by the ‘fillColor’ and ‘borderColor’ parameters related to the rectangle color.
When grouping related parameters, you also must consider if they are required or optional parameters since even if they are related, required parameters should always be defined first.
class Program
{
static void CreateRectangle(int width,
int height,
string fillColor = "White",
string borderColor = "Black")
{
Console.WriteLine($"Width: {width} and height: {height}");
Console.WriteLine($"fillColor: {fillColor} and height: {borderColor}");
}
static void Main(string[] args)
{
int width = 100;
int height = 200;
string fillColor = "Red";
string borderColor = "Blue";
// call the function with related parameters together
CreateRectangle(width, height, fillColor, borderColor);
}
}
This produces the following output:
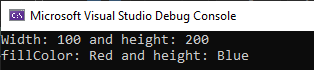
Conclusion
When defining parameters for a function, its ordering is important to preserve the programs’ consistency, clearness, and readability.
This article explained your best practices to get the appropriate parameter ordering.
First, use common sense and order the parameters to feel more natural to the method caller. Another way to order parameters is by considering their importance. If the function contains a mix of mandatory and optional parameters, required parameters should always come first.
Also, group related parameters together so that method callers can easily specify the values for the function arguments.