So, you want to unit test?
Okay, let’s get one thing straight: unit testing is not for everyone.
It’s a bold way of writing code. You might not get that instant gratification that you get from just writing some code and seeing the magical “Hello World” on the screen in a few seconds.
But unit testing is one of the most popular software practices that companies use today as part of their development cycle. This practice ensures that the code provides the desired functionality, and that is maintainable and readable.
Unit tests can both be a benefit and a hindrance to the development process and the product’s success. And this article covers the pros and cons of unit testing.
What is Unit Testing?
Unit testing is a type of automated testing. In this process, you run a single, small portion of your code, check the results, and make sure the results are what you expect. For example, you can use unit testing to verify individual classes, simple functions, or anything else of small size. It works by isolating the small chunk of code to be tested. Unit testing is a white box testing technique.
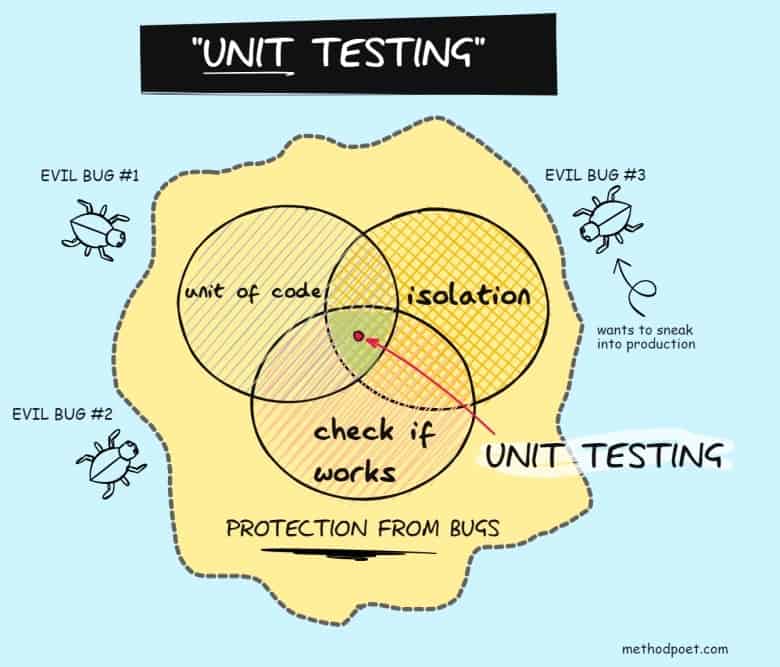
Unit Testing is an integral component of the testing pyramid. The pyramid states that unit tests are the most basic form of testing that serves as a foundation for other types of testing. However, unit testing is not meant to find the existing bugs but rather to prevent them.
The unit testing technique has many advantages.
What are the advantages of unit testing?
The unit tests have several advantages:
- It helps you write better code.
- It helps you catch bugs earlier.
- It helps you detect regression bugs.
- It makes your code easy to refactor.
- It makes you more efficient at writing code.
Let’s break down each point individually.
Unit testing helps you to write better code
Unit testing forces you to write better code.
How?
Well, to test your code, you need to make the classes testable. That means that you should use dependency injection to provide your classes with the dependencies that are needed.
It also signals you when the class is too big. When you have difficulty setting up your class in a test case, this is one of the clues that the class is too complicated. You should consider splitting the class into several smaller classes or offloading some functionality into other classes.
Unit testing helps you catch bugs earlier
In software engineering, developers test the code they’ve written before release to confirm it does what it is supposed to do. Unit testing is a type of testing where a developer tests a function or program by breaking it into small parts and testing each to see if it does what it is supposed to.
If the app doesn’t work as it should, the developers can go back and fix the problem before releasing it. That means the app gets tested during the development, thus reducing the time QA needs to spend testing the feature.
The significant advantage of unit tests is the tight feedback and test automation. Unit tests can offer feedback sooner than other tests. As a result, a developer can often perform them quickly and automatically. Unit tests are capable of providing feedback every time there is a code change. This means less back and forth with manual regression testing.
Unit testing helps you to detect regression bugs
Did you know that testing your code can help you find regression bugs? You may be writing a lot of code and forgetting to test it. This is a mistake, and you should make sure to test it before you deploy it.
What is a regression bug? It is a bug that gets introduced during the development of the application and is not found until it is released or used in production. The idea behind unit testing is to write code so that it is easy to test and hard to break. This means that if the code has been unit tested, it is easy to know if something has broken when you add new code or fix/change code.
Unit testing makes your code easy to refactor
All of us like to ship. We like to ship that new feature that we’ve been working on for the last few weeks, that change that will shave a few minutes from our e-commerce checkout process, or that few lines of code to fix a customer’s problem. Of course, we all want to ship good code, but good code comes from good testing.
Unit testing is a way to do just that.
By creating small tests for your module, you can quickly know the behaviors of the code. Unit testing also lets you refactor code more efficiently. If you have a test that proves that a specific function is working, you’ll know if you break something in the process. Unit tests give you extra confidence that you can different refactoring techniques to your code and make it better.
Unit testing makes you more efficient at writing code
Unit testing can make you more efficient at writing code. When you build, run, and fix your code, you can more quickly find and fix problems because you are testing your code as you write it.
Working on small, discrete sections of code at a time helps you identify and fix problems. In addition, your workflow becomes less frustrating with unit testing because you don’t have to worry about everything at once; you work on tight code sections one day at a time.
When there are errors, the error messages tell you exactly where the problem is. Unit testing can be tedious work, but most developers find that their code is more robust and spend less time debugging.
What are the disadvantages of unit testing?
However, unit testing also has some disadvantages:
- It takes time to write test cases.
- It’s difficult to write tests for legacy code.
- Tests require a lot of time for maintenance.
- It can be challenging to test GUI code.
- Unit testing can’t catch all errors.
Let’s break down each point individually.
Unit testing takes time
Unit testing is a very time-consuming process. It can take a lot of time to develop even one small unit test, not to mention the larger ones.
Developers need to spend time on experimentation, research, figuring out how the test method should be structured, and what methods, parameters, and objects the test case needs. It’s easy to spend an entire day on one single unit test. Not only is time spent creating the unit test itself, but also it takes time to figure out the best way to use the unit test.
Unit testing is difficult for legacy code
Unit testing legacy code is complex because it is often unclear where to begin or how to have confidence that tests are practical. To have a clear idea of where to start when unit testing, it is essential to have a clear idea about what parts of the code need to be tested. Unfortunately, it is often difficult for a developer unfamiliar with the legacy code to develop these ideas.
The second major obstacle is the structure of the legacy code.
Legacy code is messy. It is a mess that programmers must go through and tame. The code can be so tangled and chaotic that it may be impossible to write a single unit test case. Different ways of programming most likely cause code with such a messy structure. Programming that loves singletons, big classes, even bigger methods and hates dependency injection.
Unit testing requires a lot of time for maintenance
Unit testing can be time-consuming and labor-intensive. One of the biggest misconceptions is that time and labor are only required when you initially create the unit test. Suppose your team is like most, so you need to continuously test complex and interactive system. In that case, you and your colleagues will need to spend a significant amount of time and effort on an ongoing basis to maintain tests.
How much time and effort will be required will depend on your code. It’s also worth considering how extensive your testing needs to be.
Unit testing is difficult for GUI code
When developing GUI code, one of the most important things to do is test the code to ensure it works as it should. Unfortunately, unit tests are not the best way to test the GUI code. This can be due to the complexity of GUI code, how the GUI interacts with the system, or even just the idea that GUI code doesn’t code that you can test.
Unit testing can’t catch all errors
Unit tests, which test individual components of a software system, will catch many errors, but they can’t catch all of them. For example it can’t catch integration bugs. Integration bugs are the bugs that occur when you combine several units of work. To catch an integration bug, you need an integration test.
Testing software is key to reducing the number of bugs in a system. To do this, you should have a strategy for testing your software from many different angles. That means you should use different functional tests and non-functional tests to eliminate most of the errors:
- integration testing
- system testing
- acceptance testing
- stress testing (also known as load testing)
- penetration testing
Conclusion
Unit testing is an essential practice in software development to detect defects in the software in the early development stage to save time and cost.
Advantages of unit testing are that it reduces or prevents production bugs, increases developer productivity, encourages modular programming.
Disadvantages are that it is time-consuming, can’t be challenging to cover all the code, and won’t catch all bugs.
But overall, unit tests are a great way to eliminate most of the bugs now and in the future. That’s why you should use it in your day-to-day work.