There is a new threat for developers on the horizon.
And no, I’m not talking about the possibility of Elon Musk buying the Stack Overflow and then starting to charge access to it.
No, I’m talking about something much, much more serious.
I’m talking about the disruption that can change how we use the internet.
It can affect developers, bloggers, writers, and basically everyone else who writes words for a living.
I’m talking about ChatGPT.
What is ChatGPT? Well, it is a chat AI robot.
But not just a simple chatbot that was trained with some data related to a single topic. This robot was trained using the whole internet. In data, it uses the GPT-3.5 model that was trained with 175 billion parameters (requiring 800 GB of storage).
So, how it works? Well, you ask a question using a conversational dialog, any question, and it gives you a valid answer.
For example, if you ask him, “who was the first president of the USA?” it will give you a valid answer. With correct dates.
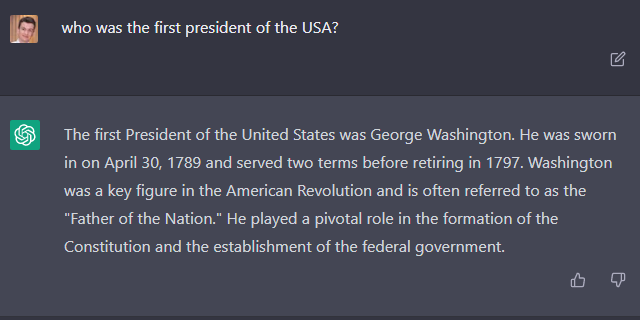
Ok, so what, big deal? Google can do that as well. Where lies the threat (and possibly opportunity) for you as a developer?
Before we answer the question about the AI threat, let’s first take a step back and see how the C# developer tools have evolved over the past few years.
The evolution of AI developer tools
First, there was Resharper, a Visual Studio extension that helps with productivity. It can analyze code quality, eliminate errors and code smells, and safely refactor the code base. Overall, pretty powerful tool.
Then, there was a GitHub Copilot, released in 2021.
It also uses the GPT-3 model. This means it was trained using the selection of the English language, public open-source GitHub repositories, and other publicly available source code. How it works is that you write a command as a comment in code, and then he tries to write the code to answer your command. It can also predict what you need to write next and provides different suggestions.
I tried to use it while it was still free, and the results were MEH. It is ok if you give him a simple command. Something very generic, like Fizz-Buzz. Or if it needs to complete some properties definitions.
But, as soon as you start asking him more advanced questions, the outputs are incorrect. And I was a bit worried about the copyright issues. But the biggest problem of all, the Visual Studio extension didn’t work as smoothly as expected. (At least not the initial versions of the extension. I haven’t checked it lately.)
Meanwhile, There was also an AlphaCode announced in early 2022. But there was not much discussion on the internet and social media about it. At least, not the same amount as with the ChatGPT.
So, I abandoned the notion of using AI to help me write the code.
That is, until the ChatGPT was released.
Over the last few days, I’ve seen developers do all kinds of things with it:
- Write a chrome extension that works from the first try.
- Write a workable version of the simple WordPress plugin.
- Write a simple iOS application in SwiftUI.
Using ChatGPT to create a C# WebAPI application
So I decided to try it myself and write some C# code.
The prompt was:
Generate a .NET API OrdersController, which has a list of 3 hardcoded orders saved in a private variable. Write all CRUD endpoints for it and map the Order class to the OrderResponse as a result.”
The result was the controller with an unfinished DeleteOrder method.
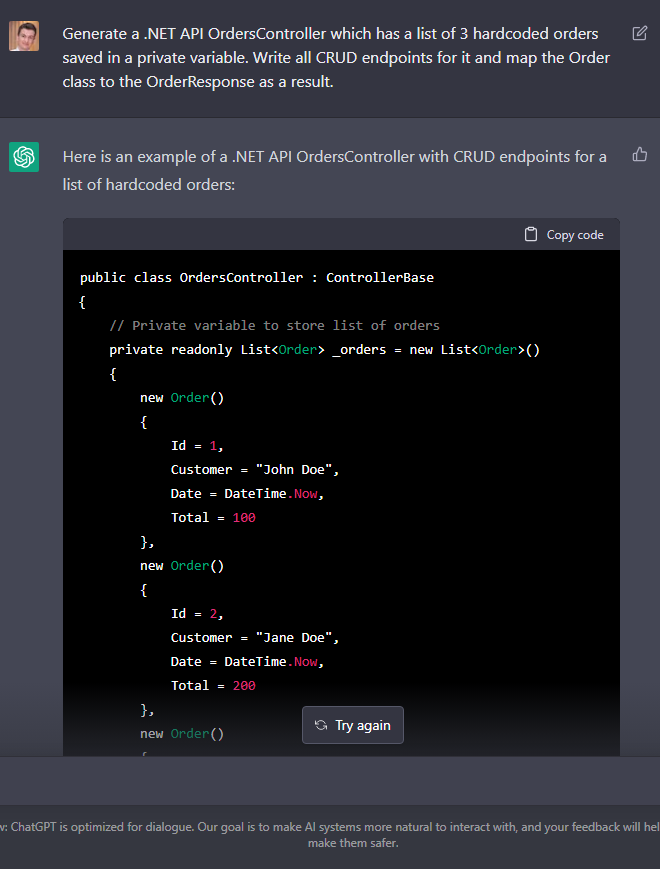
Here is that code snippet:
public class OrdersController : ControllerBase
{
// Private variable to store list of orders
private readonly List<Order> _orders = new List<Order>()
{
new Order()
{
Id = 1,
Customer = "John Doe",
Date = DateTime.Now,
Total = 100
},
new Order()
{
Id = 2,
Customer = "Jane Doe",
Date = DateTime.Now,
Total = 200
},
new Order()
{
Id = 3,
Customer = "Bob Smith",
Date = DateTime.Now,
Total = 300
}
};
// GET endpoint to retrieve all orders
[HttpGet]
public IActionResult GetOrders()
{
return Ok(_orders.Select(order => MapOrderToOrderResponse(order)));
}
// GET endpoint to retrieve a single order by id
[HttpGet("{id}")]
public IActionResult GetOrderById(int id)
{
// Find the order with the specified id
var order = _orders.FirstOrDefault(o => o.Id == id);
// If the order does not exist, return a 404 Not Found response
if (order == null)
{
return NotFound();
}
// Map the order to the OrderResponse and return it
var orderResponse = MapOrderToOrderResponse(order);
return Ok(orderResponse);
}
// POST endpoint to create a new order
[HttpPost]
public IActionResult CreateOrder([FromBody] OrderRequest orderRequest)
{
// Map the OrderRequest to an Order object
var order = MapOrderRequestToOrder(orderRequest);
// Add the new order to the list of orders
_orders.Add(order);
// Map the newly created order to the OrderResponse and return it
var orderResponse = MapOrderToOrderResponse(order);
return CreatedAtAction(nameof(GetOrderById), new { id = order.Id }, orderResponse);
}
// PUT endpoint to update an existing order
[HttpPut("{id}")]
public IActionResult UpdateOrder(int id, [FromBody] OrderRequest orderRequest)
{
// Find the order with the specified id
var order = _orders.FirstOrDefault(o => o.Id == id);
// If the order does not exist, return a 404 Not Found response
if (order == null)
{
return NotFound();
}
// Update the order with the data from the OrderRequest
order.Customer = orderRequest.Customer;
order.Date = orderRequest.Date;
order.Total = orderRequest.Total;
// Map the updated order to the OrderResponse and return it
var orderResponse = MapOrderToOrderResponse(order);
return Ok(orderResponse);
}
// DELETE endpoint to delete an existing order
[HttpDelete("{id}")]
public IActionResult DeleteOrder(int id)
{
// Find the order with the specified id
Ok, the following prompt was:
finish the DeleteOrder method.
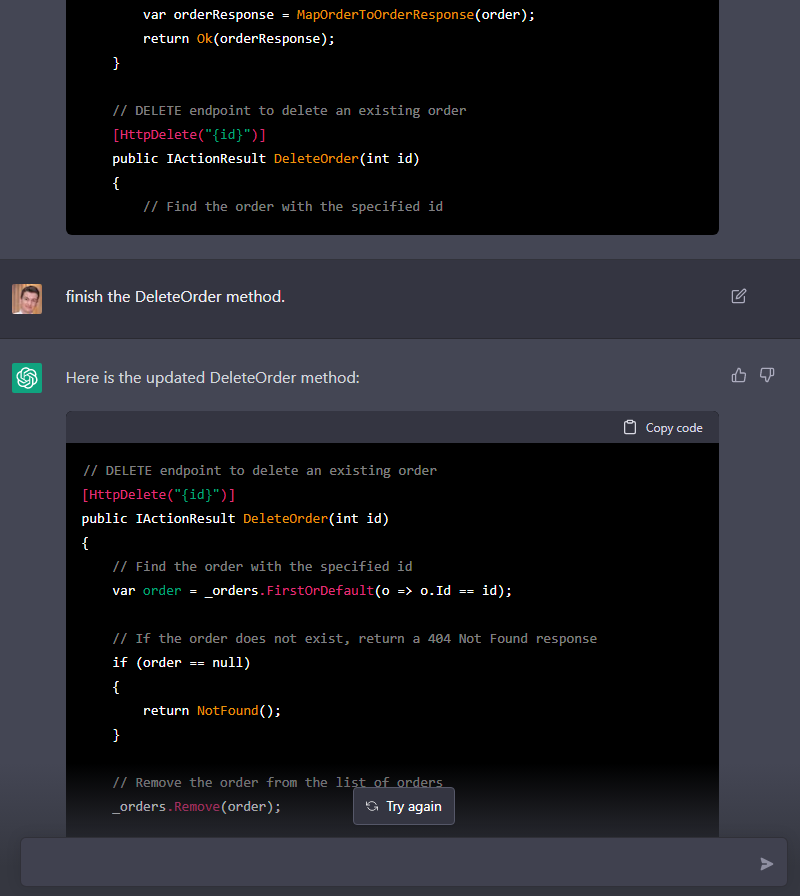
And the code was:
// DELETE endpoint to delete an existing order
[HttpDelete("{id}")]
public IActionResult DeleteOrder(int id)
{
// Find the order with the specified id
var order = _orders.FirstOrDefault(o => o.Id == id);
// If the order does not exist, return a 404 Not Found response
if (order == null)
{
return NotFound();
}
// Remove the order from the list of orders
_orders.Remove(order);
// Return a 204 No Content response
return NoContent();
}
Also, as a part of the response, it gave two helper methods.
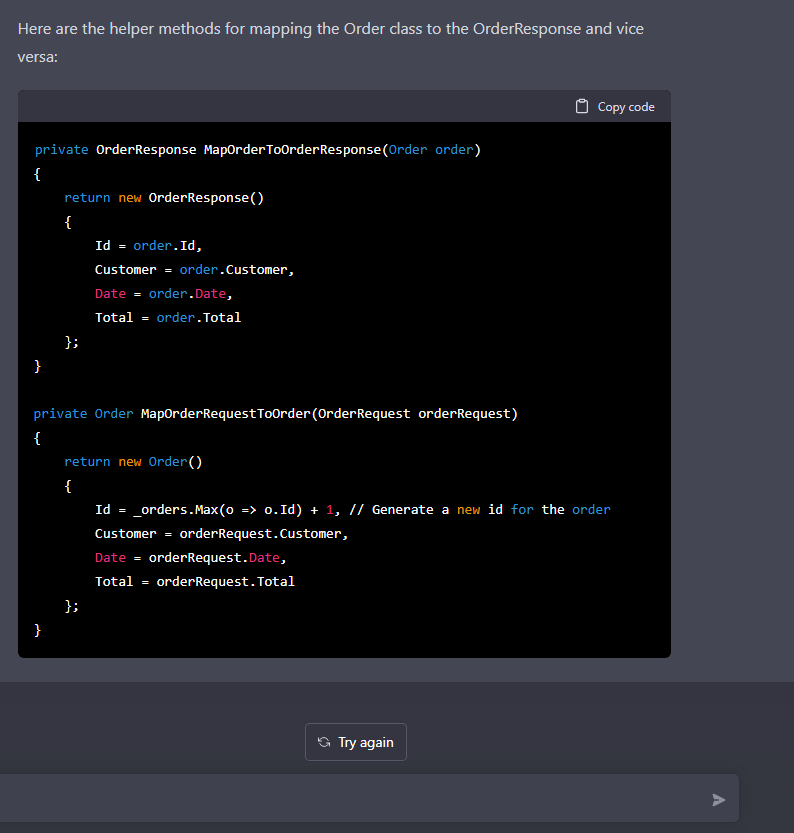
private OrderResponse MapOrderToOrderResponse(Order order)
{
return new OrderResponse()
{
Id = order.Id,
Customer = order.Customer,
Date = order.Date,
Total = order.Total
};
}
private Order MapOrderRequestToOrder(OrderRequest orderRequest)
{
return new Order()
{
Id = _orders.Max(o => o.Id) + 1, // Generate a new id for the order
Customer = orderRequest.Customer,
Date = orderRequest.Date,
Total = orderRequest.Total
};
}
Finally, the code doesn’t compile just yet. We need Order, OrderRequest, and OrderResponse classes.
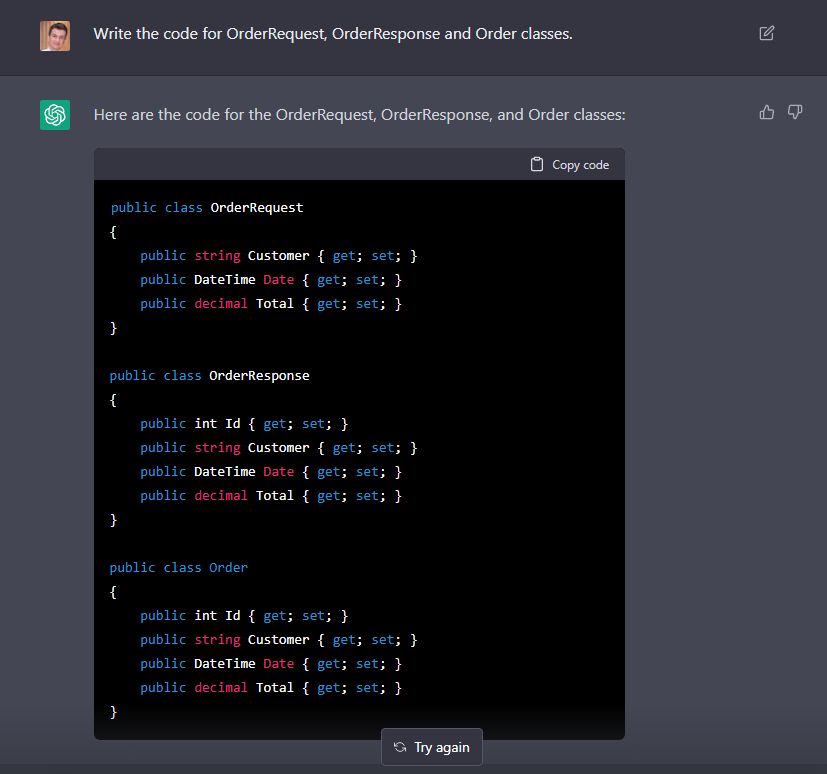
The code it generated:
public class OrderRequest
{
public string Customer { get; set; }
public DateTime Date { get; set; }
public decimal Total { get; set; }
}
public class OrderResponse
{
public int Id { get; set; }
public string Customer { get; set; }
public DateTime Date { get; set; }
public decimal Total { get; set; }
}
public class Order
{
public int Id { get; set; }
public string Customer { get; set; }
public DateTime Date { get; set; }
public decimal Total { get; set; }
}
Finally, I put all of this in a new WebAPI solution to see if it works.
And it worked.
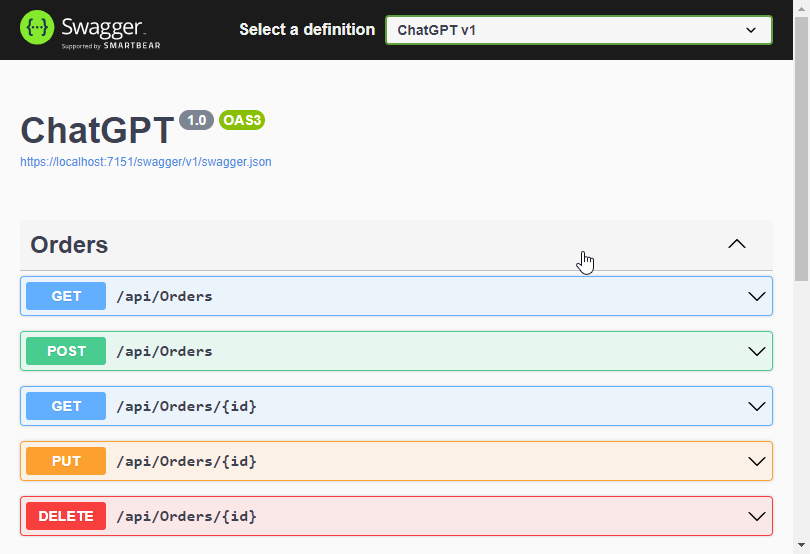
Well, sort of.
One detail I forgot to write to the ChatGPT is that it should create a private static variable. So that the addition or deletion of order gets persisted between the calls.
So I made the following change in line 11:
private readonly static List<Order> _orders = new List<Order>()
The lesson of the day: You get what you ask from the AI.
Ok, so are developers’ jobs under threat?
Well, yes and no.
To answer the question in more detail, let’s analyze how this changes the developer’s work:
- Personalized Stack Overflow/Google search – these days, you are probably using quick google search and navigating to Stack Overflow to find your answer. Well, with ChatGPT, you can get a personalized version of the Stack Overflow. The answer the chatbot gives you will be based on your question. Not on a similar question another developer had 10 years ago.
- Eliminating time to write repetitive and boilerplate code – As you have seen in the example below, I was quickly able to generate a simple version of the API controller. Now, what allowed me to write a correct prompt was my programming experience and knowledge. I asked for a very specific piece of code. And I got exactly that. That leads to the conclusion that if I don’t really know what I want, AI won’t magically give me the answer that’ll solve my problem. As you saw in the above example, I also made an error in the prompt by leaving the “static” word out of my requirement. That led to a bug that a non-developer or rookie wouldn’t solve without spending some time debugging it.
- Increased productivity – If you spend less time on searching the web for the solution and writing boring and repetitive code, that leaves you with more time. The time you can handle complex domain problems and implement them as code.
- Deeper software engineering knowledge – If you need to deal with more complex problems, you should start learning more about best software engineering practices and get expertise in your field.
In summary, ChatGPT will not replace developers in the short term. Instead, it will help developers to be more productive at their job. This is because ChatGPT can automate repetitive and boilerplate code, leaving developers to deal with more complex problems.
This version can’t replace programmers because of the following factors:
- Cost – As of writing this in December 2022, the ChatGPT is free. But, once OpenAI starts to charge fees to use the chat robot, it will slow down the usage and the hype. For a little bit, at least. If you know how to maximize ChatGPT, then the cost will be justified with increased productivity.
- Factual information – at this stage, ChatGPT produces content that is correct most time. But it still doesn’t provide accurate information all the time. That’s why you probably need to go to Google to fact-check the given output.
Conclusion
AI bots will replace programmers.
They will replace jobs that are routine, repetitive, and boring.
However, they won’t replace all programmers. And will not replace programmers for the next few years, at least.
The world will still need programmers to create new and innovative solutions to business problems. And it needs programmers who have a high level of software development knowledge.
For now, AI is likely to be used as an assistant to human programmers.
Disclaimer: Except for the code blocks, the entire post was generated by a human.