Commenting allows developers to add more context to their code.
But it’s also a tool that is easy to misuse. Lousy commenting practices often lead to code that becomes too confusing or even misleading in the long run.
Commented-out code is a familiar culprit that often appears under this category.
Why?
Well, you’ll find the answer to why the commented-out code is bad in the rest of this post.
What is the commented-out code?
Commented-out code is a source code that has been excluded or disabled from execution by adding special characters. Leaving commented-out code in source code is bad practice, as it takes up space, causes confusion, and leads to maintenance issues if it’s not removed.
Commenting out certain code blocks is a go-to practice many developers use to turn off some logic while debugging or experimenting with the code. It allows them to try out new ideas and test different assumptions without the risk of losing existing implementation details.
This in itself is not a problem.
The problem occurs when you let commented code seep into production as a part of finalized documentation. If their lifetimes extend beyond a harmless few hours, maybe days, into months and years, that’s when these comments begin to affect the code quality.
Preserving code blocks as legitimate comments in a codebase drastically reduces its readability and maintainability. The longer they remain, the worse their chance of adding meaningful context to the code becomes.
How does commented-out code affect code quality?
Most of the time, developers hesitate to remove commented-out code because:
- They think that there’s a chance it may be needed one day again.
- The removed logic in this old code may become functional again after modifying another component.
- It can offer a better way to accomplish a task if circumstances change (YAGNI, anyone?).
So, it compels developers to leave commented-out code blocks like this.
public LoginMessage PerformLogin(string username, string password)
{
if (loginAttempts > 0)
{
if (string.IsNullOrEmpty(username) || string.IsNullOrEmpty(password))
{
loginAttempts--;
return new LoginMessage("Username or password is empty.", false);
}
//else if (!allowedUsers.ContainsKey(username))
//{
// loginAttempts--;
// return new LoginMessage("Incorrect username or password.", false);
//}
else if (allowedUsers[username] != password)
{
loginAttempts--;
return new LoginMessage("Incorrect username or password.", false);
}
return new LoginMessage("Login succesful", true);
}
return new LoginMessage("No more login attempts left.", false);
}
In reality, though, these reasons aren’t a good enough reason to leave those multiple line blocks as comments in the existing code.
Source control is what you need most of the time

Often, when people try to preserve unused code as comments for the sake of “needing them in the future,” they dismiss the existence of source control.
What is source control for in programming if not for preserving history?
If you ever want to bring back an older implementation, all you need to do is go through the source code’s commit history. With a command like “git diff” or something similar, you can easily figure out what you should add to revive the old behavior.
There’s no need to maintain code as comments for months and years when source control can easily do that job for you. Commented code is rarely a better alternative to source control, especially when its downsides heavily outweigh any potential benefits.
Commented code reduces readability
One of the reasons why comments are valued in programming is how they improve code readability. But the commented-out code has the opposite effect of this intended benefit.
Whenever someone encounters a commented code block on a page, it distracts them from actual reading. Instead, it tempts them to figure out why those particular lines are commented out and what they would do when uncommented.
If the programmer cannot find answers to these questions, it can confuse them even further.
Ultimately, all this leads to the reader losing precious time trying to understand something that may not even matter in the overall context.
Commented code loses meaning over time
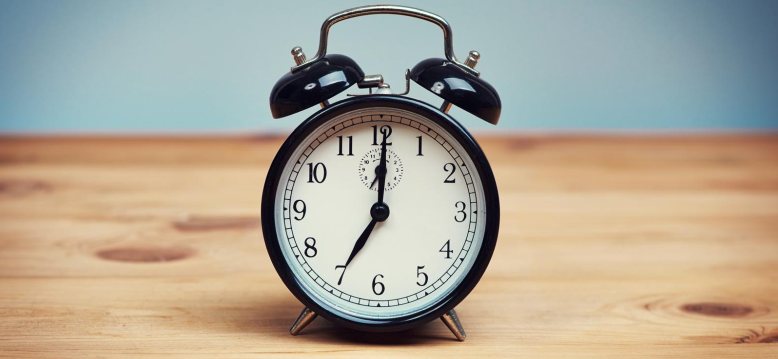
The time length that a commented code actually stays useful is relatively short.
One reason for this is related to the last point. Commented-out code snippets are difficult to understand for someone other than the person who originally commented them. Even that person can have a hard time figuring out everything after a few months.
So, in this field, where people responsible for specific features frequently change, commented code becomes more a hindrance than a help.
Now, you could say that developers can add a little more context to a commented code block using another accompanying written comment. This is true. But in addition to unnecessarily bloating the comment volume, this approach still suffers from the second reason that makes commented code useless over time.
It doesn’t take long for a commented-out block of code to become outdated or “dead”. Unlike functioning code, they are never compiled, tested, or updated as the codebase evolves over time. So, the chance of that commented block helping you resolve an issue in the future grows thinner as time passes.
In fact, it will likely cause more problems than you hope to solve in reality.
It can be a sign of a code smell
If you ever feel that keeping a certain commented-out code block is essential for one reason or another, it is most likely a code smell.
For example, consider the following instances where developers tend to use commented-out code:
- Provide more clarity to a complex logic or implementation.
- Provide an example of how to call the method/class/module.
The first instance points to an opportunity for further refactoring. If the code is too complex or ambiguous to understand without accompanying code comments, it’s probably a sign for you to break the implementation into smaller methods. Use the Extract Method refactoring, and name the method according to its intent.
The second is a responsibility that falls on documentation. It’ll require you to make the code better self-documented or provide these examples in a separate user guide. Even better, the unit test suite can provide living documentation on how to call particular methods. Either way, you shouldn’t rely on comments, something that is not regularly tested or updated, to get this task done.
Avoid checking-in commented-out code
As mentioned before, a programmer often comments code when trying to fix bugs or make changes to existing implementations. Such comments don’t cause issues as long as they stay in your personal branch, locally, while running these experiments.
But always ensure you’re not committing these comments to its remote repository, especially to a branch with several collaborators. Even though you intend to remove the comments after completing your task, it can confuse other contributors in the meantime.
It can also send a false message to other, perhaps less experienced, developers that this is an accepted way of commenting. This can have a ripple effect that leads to more and more commented code in the codebase.
There is, of course, the other possibility of you forgetting to remove these comments after completing the task.
But those comments should be identified and removed during the code review process.
You can also seek the help of linters to prevent accidentally checking in commented code.
For example, SonarLint comes with a rule that flags such comments and works with several languages, including C#. StyleCop also provides a rule that gives you a roundabout way to identify commented code on the .NET platform.
Does commented-out code affect performance?
Fortunately, commented code doesn’t have any impact on the performance of your program. Most compilers and interpreters ignore comments when processing code, leaving little chance for them to affect performance.
But as discussed above, they can definitely impact the developer’s performance when reading and modifying the code.
How should you remove existing commented code?
So, if you come across old commented-out code when working on a large codebase, what should you do? Should you remove them all at first sight? And is there a way to remove commented code in bulk?
99% of the time, the best way to deal with commented code is simply to remove them. This is even more true if understanding why the code is commented takes too much effort. There’s little chance it’ll help you or someone else in the future in a state like that. And there’s always source control if you ever want to find the block again.
But there are some rare cases where commented code might be essential. One instance is when you have a code block that should go into effect after the ongoing development/integration of another unit completes.
For example:
//TODO Opimize for speed
public void ProcessBankRequest()
{ }
These comments have a relatively shorter lifetime because you know to remove them when the expected modification happens. You can also view them in Visual Studio in the Task List window.
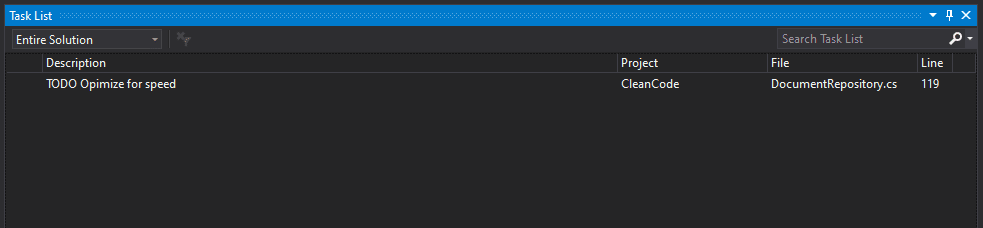
But there’s a chance that it’s something the previous developer has forgotten to clean after completing the task. So, you’ll have to understand the context of such comments before deciding on the final action.
Other times, developers use code comments to provide information on design decisions. For example, why another approach was discarded or alternative solutions.
Still, there’s a chance that you can refactor such comments to get the idea across without using commented code. Perhaps, you can change them to use pseudocode instead of explicit syntax or create separate documentation to explain the decisions.
With the majority of the commented code you come across deemed unimportant, it would be best if you had a way to remove them in bulk. Fortunately, you can find workarounds in most IDEs to achieve this goal.
In VS Code, for example, you can use its replace feature to detect potential commented-out code and delete them. A simple regular expression like “//\w+.*?\n
“ helps you identify single-line comments that don’t start with a space.
If take that a step further, use “//(\s|\w)+.?\n” to identify line comments that start with space or a string.
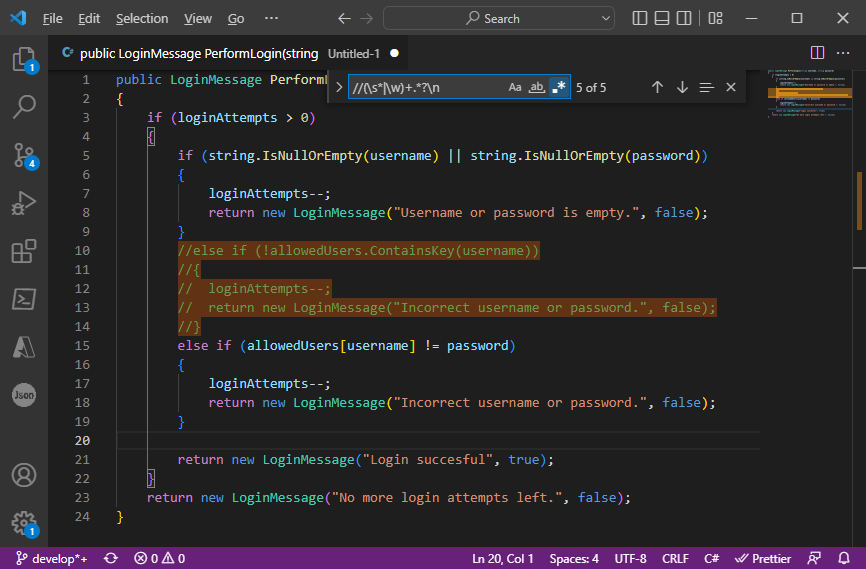
You can simply either call “replace” on a file with Ctrl+H or on the entire directory with Ctrl+Shift+H. Then, use a suitable regex to find commented code and replace them with an empty string. If you want to inspect each comment before deleting it, use “search” with Ctrl+F or Ctrl+Shift+F instead of “replace.”
What are commenting best practices in coding?
Yes, comments can be a good form of documentation. The more concise and minimalist they are, the more useful they are. Since you can’t test comments like you would test the code, too much comment quantity can burden developers.
There are some best practices you should follow while writing comments:
- Avoid noise comments
- Refactor your code when you have an explanatory comment
- Remove commented-out code
- Remove journal type of comments
- Use warning comments to explain decisions for a specific code
- Use a TODO comment sparingly
If you want to learn what each best practice means, visit the separate article on code comments best practices.
Conclusion
Commenting code is a seemingly harmless tactic developers use when modifying a codebase. But if you allow them to escape into production code, they can create a lot of headaches for future developers, and even yourself, in the long term.
Not only do they come with disadvantages that affect code quality, but they also rarely add any significant value to the codebase. While there’s a short time window where commented code can be of help, you can receive the same benefits much more easily with proper source control.
The hassle and the confusion commented code left out for too long cause is hardly ever worth whatever little convenience it brings in the short term. So, if you want to have a clean code, remove the commented-out code.