Comments in code have more than one purpose. Good comments can make code easier to understand, but it’s also important to use comments to help you maintain the code over time.
The following 6 best practices for comments in code will help you do just that.
What are the best practices for commenting code? You should:
- Avoid noise comments
- Refactor your code when you have an explanatory comment
- Remove commented-out code
- Remove journal type of comments
- Use warning comments to explain decisions for a specific code
- Use TODO comments sparingly
In this post, you will see some examples of bad and good comments. Not all comments are bad, and we will discuss that at the end of the post.
Why are comments important?
When writing code, you have to consider things like design, architecture, when to refactor, and more. However, there is another aspect of your code that can be as important for readable code, which is the comments.
Good and meaningful comments give your fellow developers insights into your code and whoever has to maintain it in the future. This is especially important if you ever move on to another job or a different project. But also during the code review process. If you stumble upon a meaningful comment during a code review, you will finish it faster.
However, comments are often not used well.
Here are the most common mistakes an inexperienced programmer commits when writing comments in code.
Noise comments
The first comments that you probably see often are so-called noise comments. This can be the comments that are not required, or that explain obvious things that can be inferred from the code.
//List of comments
private List<string> comments;
This single-line comment doesn’t add anything useful to the existing code. We have a private list of strings, and the comment is stating the obvious. This is a useless comment and should be removed.
Similarly, you can see the documentation comment on methods and properties.
/// <summary>
/// Constructor.
/// </summary>
public DocumentRepository()
{
}
/// <summary>
/// This gets/sets number of extensions.
/// </summary>
public int NumberOfExtensions { get; set; }
Again, these comments are not necessary and don’t add anything useful to the codebase.
Use method or variable instead of comment
“Don’t comment bad code—rewrite it.”
– Brian W. Kernighan and P. J. Plaugher
Many software developers use comments to leave instructions to help future developers understand the code so that the code will be more maintainable and readable. However, in many cases, comments are not needed. First, we have to ask: Do I need this comment? If it is simple logic, you can actually add a method or a variable and use it instead of a comment.
For example, if you have a comment that says, “If no employee is found, set the employee variable to null”, you can replace it with a method named “FindEmployee”.
Let’s see one code example.
public bool CheckIfCreditCanBeApproved(int amount, int age, int creditDuration)
{
//These are conditions where credit can be approved - allowed risk
if (amount < 100000 || (age < 65 && creditDuration < 15))
{
return true;
}
//other logic here....
return false;
}
We have the method CheckIfCreditCanBeApproved. At the beginning of the method, you can see the if condition and the comment above it. The comment says what are conditions where credit can be approved; so-called the allowed risk.
This comment explains the if condition. Having the quote from above in mind, let’s rewrite this code by introducing a new method.
You can easily use the Extract method refactoring inside the Visual Studio to replace the condition with the following method.
public bool CheckIfCreditCanBeApproved(int amount, int age, int creditDuration)
{
if (InputParametersAreWithinAllowedRiskRange(amount, age, creditDuration))
{
return true;
}
//other logic here....
return false;
}
private static bool InputParametersAreWithinAllowedRiskRange(int amount, int age, int creditDuration)
{
return amount < 100000 || (age < 65 && creditDuration < 15);
}
And now, you can remove the unnecessary comment.
Remember: it is a good practice to remove comments with explanatory code. This will massively improve the code quality. Just make sure you use proper naming conventions in your code.
Commented out code comments
Next, what you can often see is that you have some big chunk of code. And then, in the middle of it, you can see the part that is commented out.
public LoginMessage PerformLogin(string username, string password)
{
if (loginAttempts > 0)
{
if (string.IsNullOrEmpty(username) || string.IsNullOrEmpty(password))
{
loginAttempts--;
return new LoginMessage("Username or password is empty.", false);
}
//else if (!allowedUsers.ContainsKey(username))
//{
// loginAttempts--;
// return new LoginMessage("Incorrect username or password.", false);
//}
else if (allowedUsers[username] != password)
{
loginAttempts--;
return new LoginMessage("Incorrect username or password.", false);
}
return new LoginMessage("Login succesful", true);
}
return new LoginMessage("No more login attempts left.", false);
}
If you are working on that code, it might be a bit scary to see this multiline comment, and you just want to leave this commented code out as-is.
The right thing you should do is to remove all those comments. Find out more about why commented-out code is a bad practice here.
If you use source control such as Git, then all changes that you have made you can easily retrieve at any time. In case if there is ever a need for it. This follows a YAGNI principle.
Dear Journal… code comment
And lastly what we have is something I call “Dear journal…” code commenting practice. These types of comments explain chronologically when some kind of change occurred.
/*
* Feature 12: Added the initial calculation
* Fix 38: Remove the unneeded tax deduction
* Feature 56: Make this more generic
* Fix 112: Update constants in the method
*/
public void CalculateEmployeeSalary() { }
The block comment above represents this type of comment. If you have the CalculateEmployeeSalary method, then you can see that:
- As part of feature 12, someone added the initial calculation.
- Then we had a fix where someone removed the unneeded tax deduction.
- And so on…
These comments are not needed anymore. Before the time of source controls, this code commenting practice was a good thing to get the real picture of the changes in the code.
However, most companies and most teams use source control and you can easily browse the history of your commits and see when a particular change was made who made that change. And if the commit message is really good and has a useful description then you can get all this information.
Not all comments are bad
Okay, those were some examples of comments in code that should be avoided as much as possible. But like I mentioned at the beginning of this post, not all comments are bad.
When should you write comments and when should you just let the code speak for itself?
Sometimes the clean code alone can’t express the decision behind the implementation, and writing comments can definitely help in those situations. But remember, the best way to write good code is to understand the problem you are solving. If you can’t do that, then the writing comments won’t help either.
Warning comment
There is always some kind of comment that is useful to warn other developers in the future.

For example, we have here the method GetInfoAboutOrder. This is a private method. It has zero references which means that it should be safe to remove this method.
But if someone writes a comment: “Do not delete this method. It’s called with reflection.”, then this comment warns the other programmer that this method should be left alone.
Todo comments
If you are working on a small hobby project, you can use todo comments to know what you need to implement in the code.
Let’s see one example.
//TODO Opimize for speed
public void ProcessBankRequest()
{ }
Here is the ProcessBankRequest method. It has a TODO comment above it. In Visual Studio, if you prefix your comment with TODO, then you can use the Task List window to list all those comments in one place.
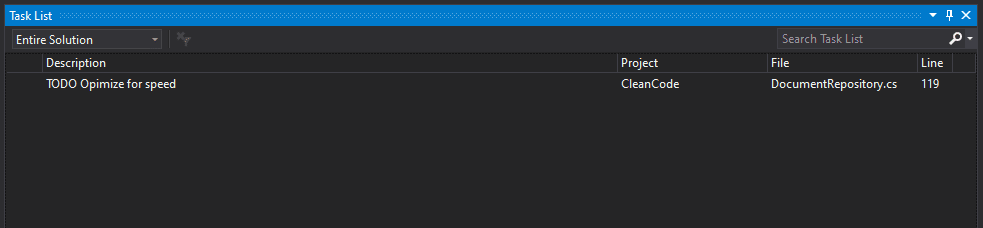
One word of caution: this comment is fine if you are working on a solo project and you don’t use a separate tool to track your tasks. If you are working on a team, don’t overuse this code commenting technique. Instead, use a dedicated project management tool for all the tasks you need to do.
Conclusion
Should you use comments in your code? Yes.
If used properly, they can make your code easier to read and maintain. However, if misused, they can have the opposite effect. This post gave you a quick overview of the types of comments, their best practices, and some common mistakes.
Comments can be an important part of a project. They’re a way to provide context and explain the purpose of various lines of code. They can also serve as a way to document your code. But based on my experience, a better way to provide technical documentation is through well-named unit tests.
You do use unit tests, right?