Do you struggle to create maintainable code in C#?
Do you feel like you are constantly reinventing the wheel while trying to solve problems that lead to messy code?
If you do, don’t worry. You are not alone.
Many developers experience the exact same thing.
Luckily, there is a light at the end of the tunnel.
Meet design patterns in C#.
What we call “design patterns” in the software industry are answers to the kinds of software design issues that repeatedly occur during real-world application development.
When we talk about patterns, we’re talking about code templates in object-oriented programming that you can use repeatedly.
A design pattern is a standardized way to describe how to fix an issue that may occur in various contexts.
This article explores the 23 design patterns available in C#.
You will get an overview of every design pattern with a link to a separate article explaining a particular pattern in more depth. You will also learn why they’re important when developing software.
Are you ready? Then let’s go!
What are design patterns?
A design pattern is a repeatable solution to a commonly occurring problem in software development. A design pattern isn’t a finished design that you can copy and paste directly into code. Instead, it is a description or template for solving a problem that you can use in many different situations.
Design patterns provide detailed solutions that you can apply in real-world scenarios. In addition, they are written in specific language terminology so developers can easily understand them with different experience levels. That’s why you can also use them as a communication tool when discussing design decisions and architectural issues with others.
As a result, you don’t have to reinvent the wheel when encountering a common software design problem. Instead, you can use an existing design pattern to save time and effort.
In 1994, Erich Gamma, Richard Help, Ralph Johnson, and John Vlissides, also known as the Gang of Four, compiled a list of 23 design patterns and published them in the Design Patterns: Elements of Reusable Object-Oriented Software book. The book became a bestseller, read by millions of developers worldwide, and an all-time classic.
Another book related to design patterns that is very popular is Head First Design Patterns. It explains the design patterns in a very casual and interesting way. So I would definitely recommend reading that book as well, in addition to the original Design Patterns book.
What are the 3 types of design patterns?
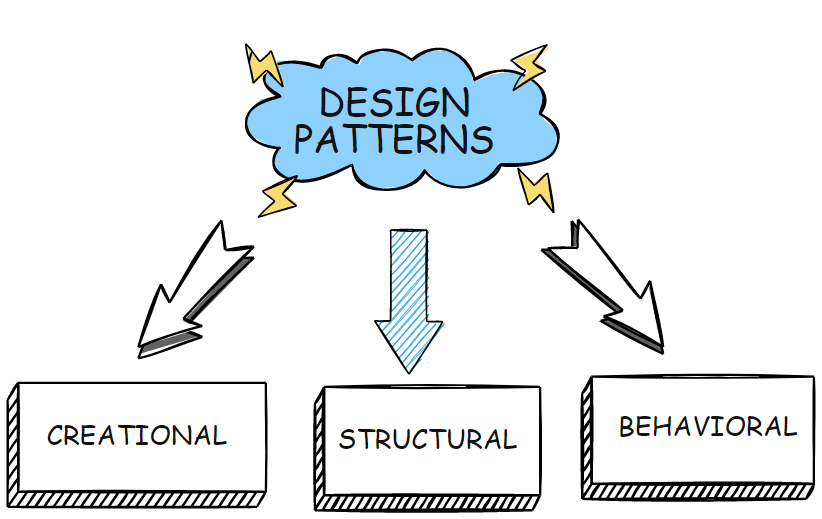
There are three main categories of design patterns:
Creational design patterns
Creational design patterns address issues occurring while making new objects. They aid in hiding the complexity of object creation.
The 5 patterns that follow are all examples of creational patterns:
- Abstract Factory design pattern – provides an interface for creating a group of factories with a common use case without specifying the concrete classes. Each concrete implementation of the abstract factory creates concrete objects that are part of the same family of products.
- Builder design pattern – can create a complex class in a step-by-step manner. It is useful when constructing a complex object requires many different steps or when the order of those steps is important.
- Factory Method design pattern – defines a method for creating an object but allows subclasses to determine which object should be created. The type of the instantiated object can be determined during runtime.
- Prototype design pattern – which you can use when a prototype instance determines the type of object you need to create. Then, that prototypical instance is cloned to create new instances of the same type.
- Singleton design pattern – allows you to create only one instance of a class and gives you global access to it.

Structural design patterns
Structural design patterns solve the problems in relationships between different classes and objects.
If you need to improve the structure of your code, consider one of the 7 following structural patterns:
- Adapter design pattern – allows two software components to work together even if they have incompatible interfaces.
- Bridge design pattern – is used to decouple an abstraction from its implementation. As a result, the abstraction and implementation can be developed and changed independently.
- Composite design pattern – allows for the treatment of individual objects and compositions of objects in the same way.
- Decorator design pattern – allows you to add behavior to an existing class without modifying the original code.
- Facade design pattern – provides a unified interface to a set of classes, hiding their complexity from the client code.
- Flyweight design pattern – describes how to use the objects efficiently to reduce the cost of operations.
- Proxy design pattern – uses a single object, called a proxy, to act as a substitute for a specific class.
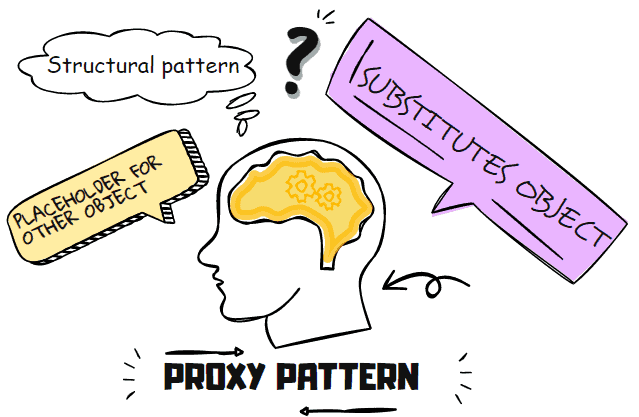
Behavioral design patterns
Behavior design patterns provide solutions to common problems regarding interaction between classes and objects.
Use one of the following 11 behavioral patterns to improve how objects communicate in your application:
- Chain of Responsibility design pattern – allows an object to send a request to a chain of other objects to find the object that can best handle the request.
- Command design pattern – encapsulates a request as an object, thereby allowing for the parameterization of clients with different requests and providing a historical record of requests.
- Interpreter design pattern – defines a grammar for specifying a language and interpreting sentences in that language.
- Iterator design pattern – allows you to define an interface to access the elements of complex collections sequentially while hiding their representation from the client.
- Mediator design pattern – allows loose coupling between objects by encapsulating how these objects interact. It eliminates the need for these objects to communicate directly with each other, reducing the system’s overall complexity.
- Memento design pattern – creates a snapshot of the internal state of an object at any instant of time and makes it available for access externally.
- Observer design pattern – is a software design pattern in which an object, called the subject, maintains a list of its dependents, called observers. When the subject changes state, it notifies its observers.
- State design pattern – allows an object to change its behavior based on its internal state. The pattern is used to create objects that represent various states and transitions between those states.
- Strategy design pattern – gives flexibility in deciding which algorithm to use during runtime. This is useful when an application uses a family of algorithms for different scenarios or when the strategy needs to be dynamically selected at runtime based on input data.
- Template Method design pattern – defines the skeleton of an algorithm in a superclass but lets subclasses override specific algorithm steps.
- Visitor design pattern – is a pattern where a visitor object is used to perform operations on other objects. Use the visitor design pattern when adding new operations to an already existing object.
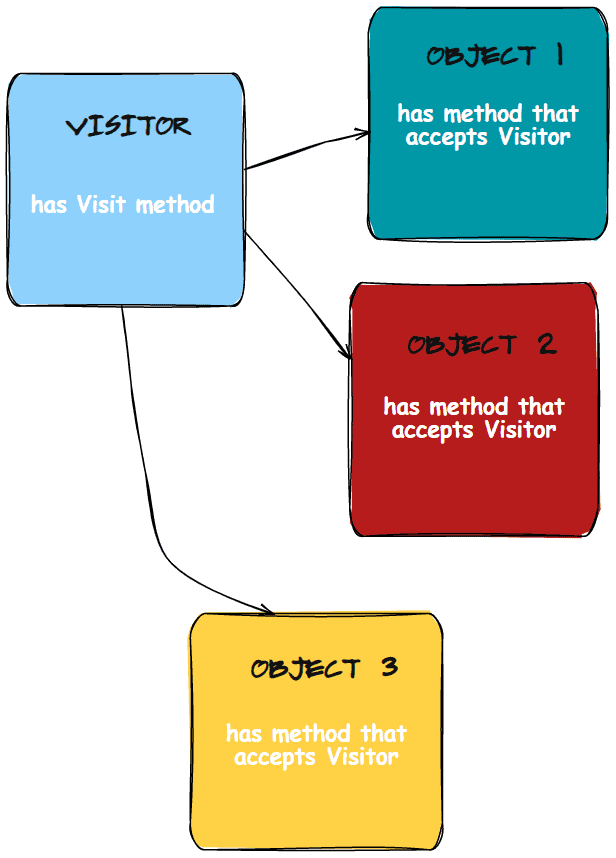
Why do we use design patterns?
The purpose of design patterns in C# and other high-level programming languages is to solve problems that arise repeatedly. This is done by identifying the optimal balance between speed, simplicity, and complexity in generating a solution that addresses all those issues simultaneously. A design pattern is a proven template and a reusable solution for reoccurring problems.
To be more precise, deciding to use a particular pattern in C# is not limited to resolving a single issue. Instead, due to their efficiency, patterns can address a wide variety of issues with only a small amount of code.
Just to be clear: design patterns add some complexity. But, on the other hand, they usually solve a much bigger problem, so they are well worth the solution if you apply them correctly.
Plus, nowadays, most programmers have a solid understanding of design patterns. In other words, they are aware of the tool’s potential. So, by adding a design pattern to a source code, you clearly communicate how you have decided to solve a problem.
Are design patterns still used?
With the rise of microservices and cloud computing, you might wonder if design patterns are still a thing.
And the answer is yes.
Design patterns are still used in modern application development since they provide a way to structure the code in an organized and efficient way. They make development easier and faster in the long run. Design patterns have been used since the early days of software development, and they continue to be used today.
Even if you have an application that uses microservices and cloud computing, you will likely still need to implement design patterns to improve your code design.
Moreover, many popular frameworks and libraries implement many design patterns.
Let’s take, for example, MediatR, a lightweight and flexible library that makes it easier to create a mediator and communicate between your services and your business logic. It uses a combination of the Mediator and Command design patterns.
Even if you are stuck with a legacy application, you can probably eliminate some mess with an appropriate design pattern.
By understanding how to use design patterns effectively, you create better products that are more robust and maintainable over time. That’s why getting familiar with them can help you create better products that are more robust and maintainable over time.
Why learn design patterns?
Design patterns created by seasoned object-oriented practitioners may make your projects more adaptable, robust to change, and manageable. If design patterns aren’t already a part of your development arsenal, here are three good reasons to start using them.
#1 Avoid adding unnecessary complexity by trying to solve problems with whole new solutions
There is one thing that always remains the same in software development projects. And no, I’m not talking about the fact that projects are constantly late and over budget.
I’m talking about change.
Software architecture and structure of classes change all the time due to the:
- change in requirements,
- new features being introduced,
- performance enhancements.
But with changes come new problems.
So the challenge for developers who want to have a good design in code is to lessen the effects of constant changes. That’s why learning about how to apply design patterns properly can avoid adding unnecessary complexity by trying to solve new problems with new solutions.
#2 Hone your object-oriented abilities
By using abstraction, inheritance, polymorphism, and encapsulation through design patterns, you can eliminate issues like code duplication, brittle design, and an uncontrolled increase in the number of classes.
Not only do they provide best practices for object-oriented programming, but they also help to improve your coding skills and make it easier to understand complex systems.
So if you want to develop your skills in object-oriented design and create flexible and easily maintained applications, then learn design patterns.
#3 Rely on the strength of a common vocabulary
One of the advantages of studying design patterns is that you and your team will get additional power if you are all acquainted with common patterns. Using design patterns improves developers’ communication by allowing them to discuss issues using a shared vocabulary.
Design patterns provide a common language that you can use to talk about the structure and behavior of software applications.
As a result, developers can communicate more effectively with each other. They also help you understand existing code. For example, if you see a class whose name ends with “Builder”, you know that the class intends to create an instance of the class in a step-by-step manner.
Should you learn all design patterns?
Ideally, yes, but if you don’t have time right now to study all of them, here is my advice.
Now, there are 23 design patterns. But we can be a little bit pragmatic here. Out of those 23 patterns, you will probably 80-90% of the time use one of these 7 patterns:
- Factory Method
- Builder
- Adapter
- Decorator
- Facade
- State
- Strategy
If even that’s too much for you, just learn Factory Method, Builder, and State.
But as I mentioned, you should invest your time learning all design patterns because you can use that knowledge in any project and any programming language. So the return on your investment of time is enormous.
Are design patterns hard to learn?
Design patterns require learning time, but most are not hard to understand. But the key is not to learn how to implement a design pattern. Instead, the key is understanding each pattern’s purpose and how you can apply them to different projects. And the rewards of learning them are well worth it.
With a better understanding of design patterns and with the help of practice, you will find that understanding these principles will help them craft better code in a shorter amount of time.
If you think about design patterns in another way, there isn’t much originality in most of those patterns. They’re simply fancy names for object-oriented composition that makes sense.
What about SOLID principles?
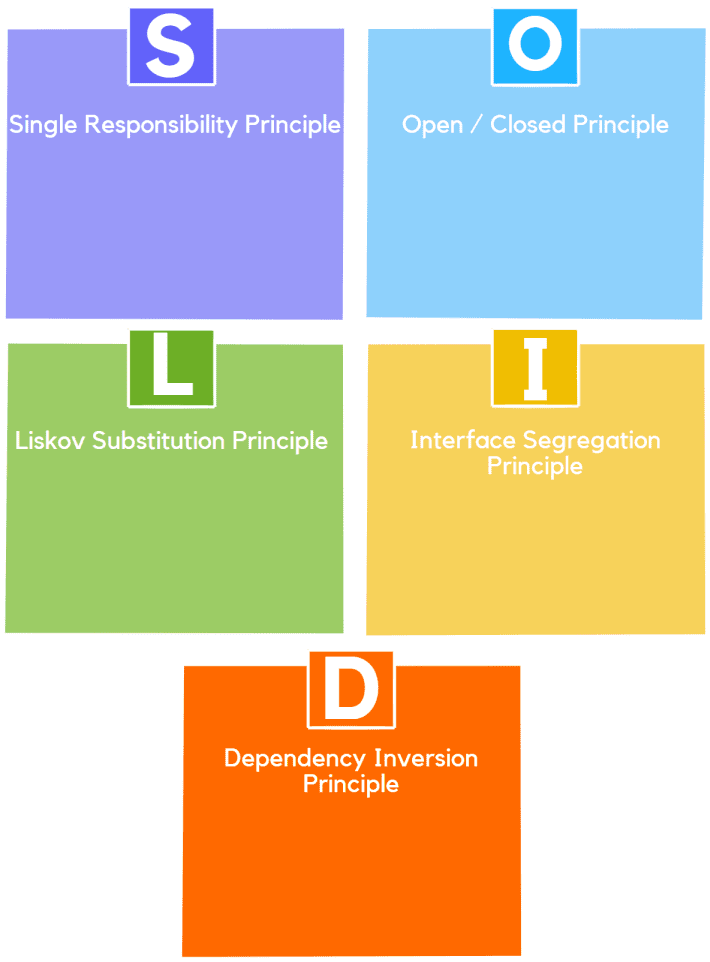
When developers talk about learning design patterns, the topic that also arises is SOLID principles.
So, what are SOLID principles, and how are they different from design patterns?
The SOLID principles are a set of principles that guide software developers in their development processes. They offer guidelines and advice on how to craft better software.
The acronym stands for:
- S – Single Responsibility Principle (SRP)
- O – Open/Closed Principle (OCP)
- L – Liskov Substitution Principle (LSP)
- I- Interface Segregation Principle (ISP)
- D – Dependency Inversion Principle (DIP)
Robert C. Martin first introduced them in his paper Design Principles and Design Patterns.
The difference between design patterns and SOLID principles is that design patterns are reusable solutions to common code problems, while SOLID principles offer guidelines and advice on how to build better software. Design patterns provide a specific code template, whereas SOLID offers more general advice.
Conclusion
Design patterns play a pivotal role in deciding the best course of action when finding answers to challenges that demand maximum effectiveness and forward development at every stage. They aid developers by providing a battle-tested solution and may solve a wide variety of common problems in C#.