I’ve been a programmer for about a decade now.
And one thing that’s always frustrated me is the methods that have five, six, or even more parameters. You know what I’m talking about: it’s like the developer is trying to throw everything they can at the method and hope something sticks. However, this is a bad idea because there is no way of knowing the method’s real intent.
Thankfully, there’s a solution. It’s called Introduce Parameter Object refactoring.
Introduce Parameter Object is a refactoring that you can use to replace long parameter lists with a single parameter of an object. The object is used as a container for method parameters. The advantage of this refactoring is that you can add new parameters without changing the method.
In this post, I’ll show you this hands-down best approach to reducing the number of method parameters.
What is Introduce Parameter Object refactoring?
There’s a huge debate about how many parameters a method should have.
The general rule is that a method shouldn’t have more than three parameters. This rule was first introduced in the Clean Code book. It states that, ideally, the method should have zero parameters. A method with one or two parameters is still ok, but three or more function arguments are too many.
Does this mean you should change every method in your application that has more than three arguments?
The answer is no.
We don’t live in an ideal world. And it’s hard to change every method with three parameters.
So, where is that limit? Well, if a method has five or more parameters, then you should consider refactoring that method and decreasing the number of parameters.
This is where Introduce Parameter Object refactoring comes into play. In short, this refactoring is a way of replacing a method’s argument list with a single parameter object. It’s used to simplify the code, which is something you should always do.
Interestingly, the number of parameters in a method is directly related to how often it will be called. The more often a method is called, the more parameters it will have and the more code you’ll have to write to support it.
And it’s not just the method itself that comes with more parameters; each parameter it takes will increase the number of parameters in the method. This results in a rather vicious cycle: the more often a method is called, the more parameters it has.
Refactoring steps
To refactor the multiple arguments to a parameter object, follow these steps.
- Create a new class that will represent the parameters.
- Add this new class as a parameter to the existing method parameter list. You can start by passing null to all existing calls of the method.
- Take the first parameter in the method. Pass it to the method using parameter object. And remove the first parameter after that. Repeat the above step until you remove all remaining parameters, leaving the method only with a parameter object as an argument.
Example
Let take a look at the following method.
public void SaveHomeAddress(string name, string homeAddress, string country, string email, string fileLocation)
{
if (string.IsNullOrEmpty(name) || string.IsNullOrEmpty(homeAddress)
|| string.IsNullOrEmpty(fileLocation))
{
Console.WriteLine("Input parameters are empty");
}
else
{
using FileStream fileStream = new FileStream(fileLocation, FileMode.Append);
using StreamWriter writer = new StreamWriter(fileStream);
List<string> aPersonRecord = new List<string>
{
name,
homeAddress,
country,
email
};
writer.WriteLine(aPersonRecord);
}
}
This method writes a person record to the file. The method has five parameters and we want to replace it with the parameter object.
We start with creating a new class, called AddressDetails
.
public class AddressDetails
{
public string Name { get; set; }
public string HomeAddress { get; set; }
public string Country { get; set; }
public string Email { get; set; }
public string FileLocation { get; set; }
}
The next step is to add a new parameter to the SaveHomeAddress
method.
public void SaveHomeAddress(string name,
string homeAddress,
string country,
string email,
string fileLocation,
AddressDetails addressDetails)
And update the calls to the method with null
value as the last parameter.
SaveHomeAddress("John",
"New York",
"USA",
"john@methodpoet.com",
"C:\\temp",
null);
Now we can construct the instance of the object with the parameters.
var addressDetsils = new AddressDetails
{
Name = "John",
HomeAddress = "New York",
Country = "USA",
Email = "john@methodpoet.com",
FileLocation = "C:\\temp"
};
SaveHomeAddress("John",
"New York",
"USA",
"john@methodpoet.com",
"C:\\temp",
addressDetsils);
And start replacing parameters one, by one. Let’s start with replacing name
with addressDetails.Name
. After that, we can remove the name parameter.
public void SaveHomeAddress(string homeAddress,
string country,
string email,
string fileLocation,
AddressDetails addressDetails)
{
if (string.IsNullOrEmpty(addressDetails.Name) || string.IsNullOrEmpty(homeAddress)
|| string.IsNullOrEmpty(fileLocation))
{
Console.WriteLine("Input parameters are empty");
}
else
{
using FileStream fileStream = new FileStream(fileLocation, FileMode.Append);
using StreamWriter writer = new StreamWriter(fileStream);
List<string> aPersonRecord = new List<string>
{
addressDetails.Name,
homeAddress,
country,
email
};
writer.WriteLine(aPersonRecord);
}
}
You need to repeat this step until all other parameters are removed and only parameter object remains.
public void SaveHomeAddress(AddressDetails addressDetails)
{
if (string.IsNullOrEmpty(addressDetails.Name) || string.IsNullOrEmpty(addressDetails.HomeAddress)
|| string.IsNullOrEmpty(addressDetails.FileLocation))
{
Console.WriteLine("Input parameters are empty");
}
else
{
using FileStream fileStream = new FileStream(addressDetails.FileLocation, FileMode.Append);
using StreamWriter writer = new StreamWriter(fileStream);
List<string> aPersonRecord = new List<string>
{
addressDetails.Name,
addressDetails.HomeAddress,
addressDetails.Country,
addressDetails.Email
};
writer.WriteLine(aPersonRecord);
}
}
Other options to reduce the number of parameters
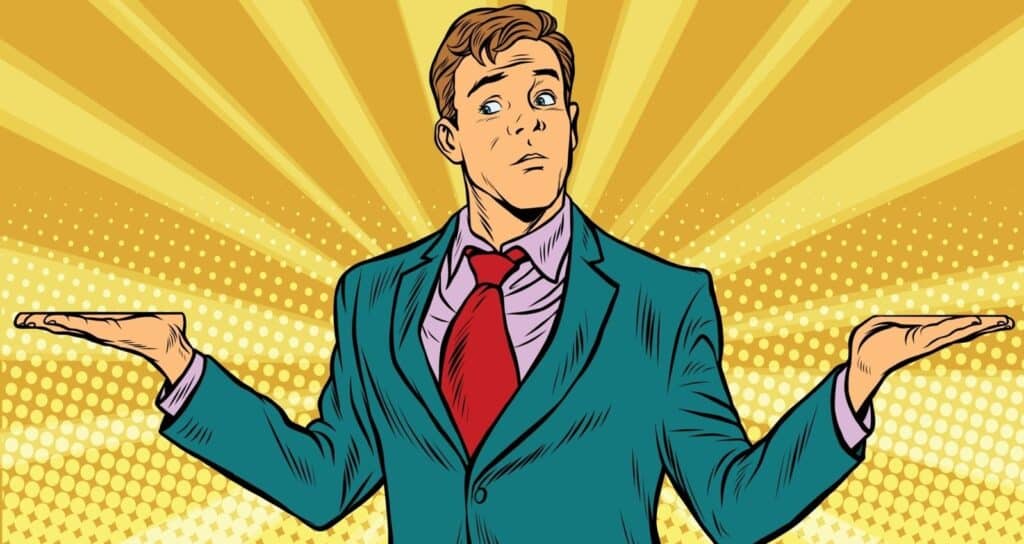
There are other options to reduce the excessive list of parameters.
Make the method itself smaller
Sometimes, methods in your code can be too long. They get too big and too cluttered. They get hard to read. They get hard to maintain. Extract Method is a way to fix this.
You can break the method into smaller methods, then call the smaller methods from the parent method. Sometimes you can then call the extracted method directly and remove this call from this original method. This can lead to decreasing the number of method parameters.
Remove unnecessary parameters
Removal of unnecessary parameters can help reduce your code size. For example, if you have a method that takes in an integer and a string and with no reason for those two parameters, you can refactor the code to remove the unnecessary parameters and provide the same functionality in a cleaner manner. The fewer parameters you have, the better off your code will be.
How to do it in Visual Studio?
It’s simple:
- Right-click on the method and select Quick Actions and Refactorings…
- Select the Change Signature… option.
- The window will present to you. In this window, you have a Remove button. Click on the method parameter you want to eliminate and click on the Remove button.
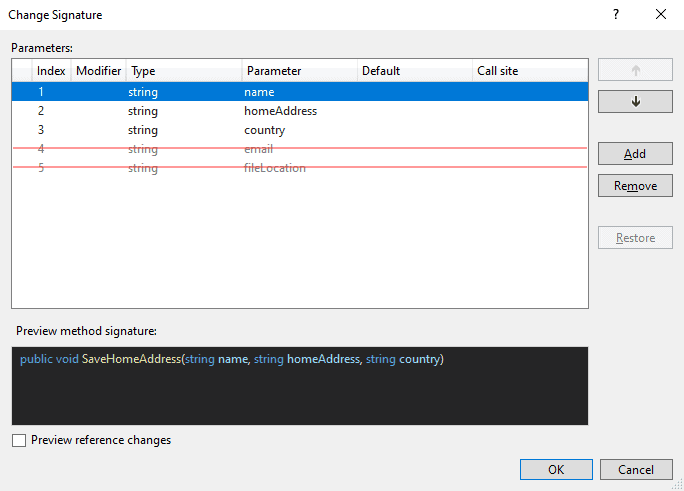
How to reduce the number of constructor parameters
Constructors can easily and quickly increase in size and complexity. It’s not uncommon for a class to take seven or eight constructor parameters. However, with increased parameters, comes the increased complexity.
To reduce the number of constructor arguments, use these techniques:
- Split the class into multiple classes
- Use the Builder design pattern
- Introduce Parameter Object
- Use the Facade design pattern
I have a separate article with code examples on using the above suggestions to make your constructors less complex.
Conclusion
Software development is all about constantly making changes to create better software. After all, the software never stands still. So, in their quest to make software better, developers are constantly refactoring or making changes to existing code.
Introduce Parameter Object is a refactoring that can help you to eliminate methods with a long parameter list. By introducing this object, the future changes to the method become easier.
You can remove parameters. You can add new parameters. The parameter object doesn’t care. It can handle it all.