If you’re looking for a job in software development, you need to be prepared to answer questions about unit testing. Unit testing is a critical part of the software development process, and employers want to know that you understand how to create and implement effective unit tests.
To help you prepare for your next job interview, here is a list of 50 unit testing questions to help you demonstrate your knowledge and expertise. These questions cover a wide range of topics, including what unit testing is, why it is important, and a little bit about other types of testing.
Whether you’re a beginner or an experienced software developer, these 50 questions will get you hired.
1. What is unit testing?
Unit testing is a type of software testing that is used to verify the functionality of individual units of code. A unit can be any small piece of code, such as a method or a class. Developers typically do unit testing while writing code to catch any errors as early as possible.
Unit testing is an important part of developing high-quality software, as it can help ensure that code works as intended and that there are no hidden bugs. It can also save time and money in the long run, as fixing bugs early on is usually cheaper than fixing them later.

Unit tests are typically written using a testing framework, which provides tools and defines how the tests should be structured.
Further reading about the basics of unit testing.
2. What is the arrange-act-assert pattern?
The arrange-act-assert pattern is a methodology used in automated testing. It contains three steps: arrange, act, and assert. In the arrange step, the test data and test environment are set up. In the act step, the test is run. Finally, in the assert step, the test results are verified.
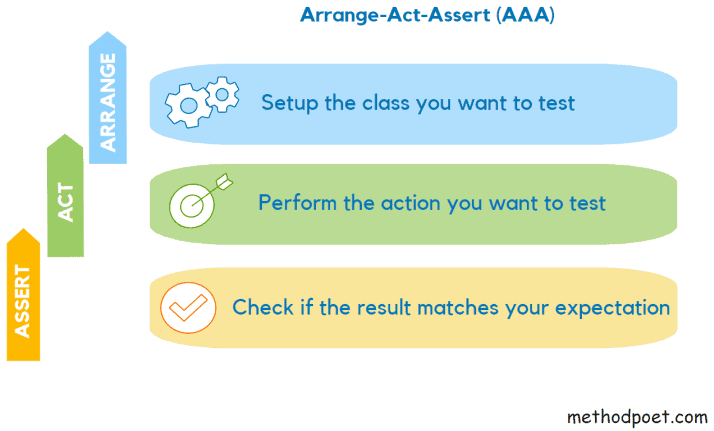
Further reading about the arrange-act-assert pattern.
3. What is a fake?
When it comes to unit testing, there are a few things to keep in mind:
- Unit tests should only test a single piece of functionality.
- All unit tests should be completely independent of each other.
- All unit tests should be deterministic, meaning they should always give the same result when run on the same input.
There are many ways to answer this question, but ultimately it boils down to this: a fake is anything that allows you to isolate the behavior of the unit you are testing from its dependencies. For example, this could be a mock object that simulates the behavior of a real object, or a stub that simply returns pre-determined values.
4. What is a stub?
In software development, a stub is a piece of code that emulates the behavior of a real component. For example, stubs are used in unit testing to provide a known, controlled environment to test a target component’s behavior.
For example, if you are testing a component that makes an HTTP request, you would use a stub to simulate the HTTP server. The stub would return a known response, so you could test how your component handles that response.
You can also use stubs to simulate error conditions. For example, you might stub a component to always return an error, so you can test how your component handles errors.
In general, stubs should be small and simple. Furthermore, they should only implement the behavior relevant to the test you are trying to perform.
5. What is a mock?
In software development, a mock is an object that simulates the behavior of another object.
You can also use mocks to verify that the unit of code under test interacts with the object or service in an expected way. For example, you can use a mock to verify that a method is being called with the correct arguments.
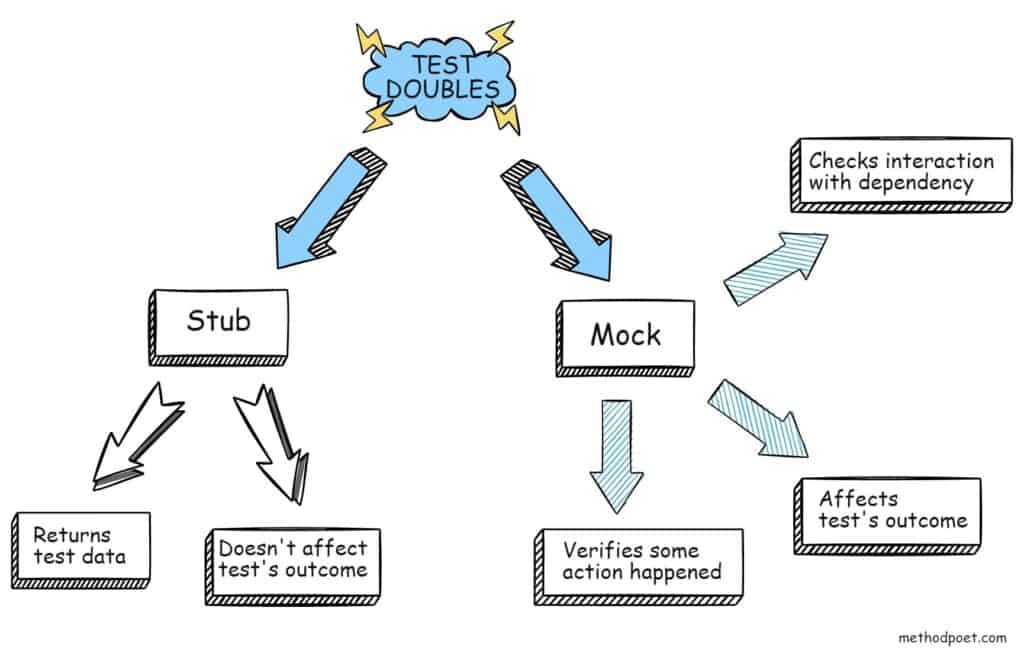
So, what is the difference between a stub and a mock? A stub only emulates the behavior of another piece of code and returns fake data. On the other hand, mock verifies that the desired behavior has happened.
Further reading about stub, mock, and their differences.
6. What is code coverage?
Code coverage measures how much of your code is being tested by your automated tests. It’s typically expressed as a percentage and can be a useful metric for determining your tests’ effectiveness.
Code coverage is not a perfect metric, but it can be a helpful tool for understanding how your tests are performing. If you have a high code coverage percentage, your tests are likely doing a good job of covering your code. However, if you have a low code coverage percentage, your tests may be missing some important parts of your code.
7. What are the advantages of unit testing?
The advantages of unit testing are:
- you have better code
- you catch bugs earlier
- they detect regression bugs
- easier refactoring
- you are more efficient at writing code.
8. What are the disadvantages of unit testing?
The disadvantages of unit testing are:
- it takes time to write tests
- it’s hard to write tests for the legacy code
- it’s challenging to test GUI code
- can’t catch all errors
Further reading about the advantages and disadvantages of unit testing.
9. What is state-based unit testing?
State-based unit testing is a type of unit testing in which the units (i.e. the individual components of a system) are tested in isolation from each other. In state-based unit testing, the focus is on the internal state of the units rather than on the interactions between them.
State-based unit testing can be more robust and reliable than interaction-based unit testing, as it is less likely to be affected by changes in the system’s environment or how the units interact.
10. What is interaction-based unit testing?
In software engineering, interaction-based testing is a technique for testing the behavior of a system by exercising its collaborations. That is its interactions with other systems.
Interaction-based testing focuses on specifying and verifying the behavior of a system in terms of its interactions with other systems. It is beneficial for testing systems that interact with other systems in complex ways, such as distributed systems.
Further reading about state-based and interaction-based testing.
11. Who performs unit testing?
Unit testing is vital to creating high-quality software, and the developers usually write and maintain unit tests. However, there are some cases where a separate team of testers performs unit tests. This is often the case in large organizations where the developers do not have time to write and maintain the unit tests themselves. Unit tests are essential to the quality assurance process in these cases.
Further reading about who performs unit testing.
12. What is exploratory testing?
Exploratory testing is a type of testing that is conducted without pre-determined test cases or scenarios. This type of testing allows testers to discover new areas for testing and better understand the system under test.
Exploratory testing is often used with other types of testing, such as manual or automated testing. When combined with these other types of testing, exploratory testing can help find more bugs and get a more comprehensive understanding of the system under test.
You should keep a few things in mind before considering using exploratory testing in the software testing process. First, exploratory testing is not a replacement for other types of testing. It should be used in addition to other types of testing, not instead of them. Second, exploratory testing is best conducted by experienced quality assurance engineers.
13. What is white-box testing?
White-box testing is a type of software testing technique that looks at the internal code structure of a software program to find errors. It is also known as clear box testing, glass box testing, logic-driven testing, and structure-based testing.
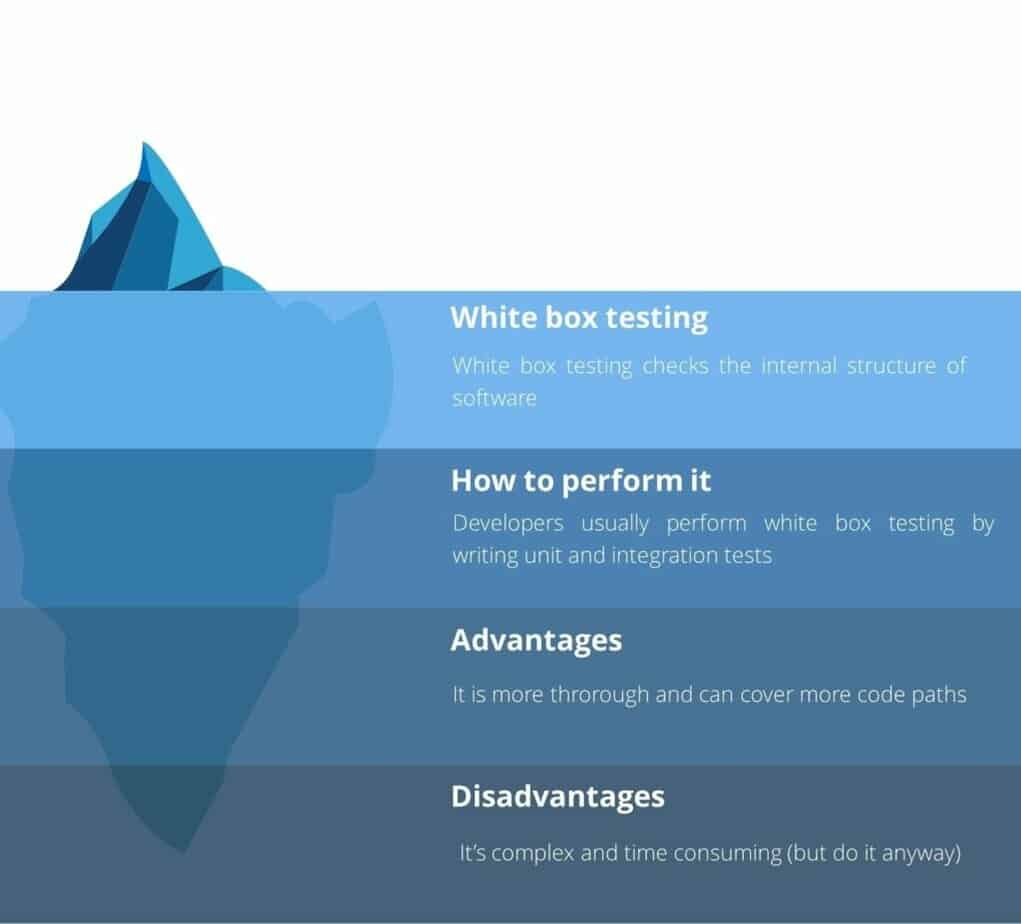
White-box testing is generally considered more thorough than black-box testing, as it allows testers to examine the code directly and thus have a better understanding of how the program works. This can be especially useful for finding errors in complex programs. However, white-box testing can be more time-consuming than black-box testing, as it requires knowledge of the code structure.
14. What is black-box testing?
Black-box testing is a method of testing software where the tester does not have access to the program’s inner workings. In other words, the tester is only given the inputs and outputs of the program and must figure out how it works based on that information.
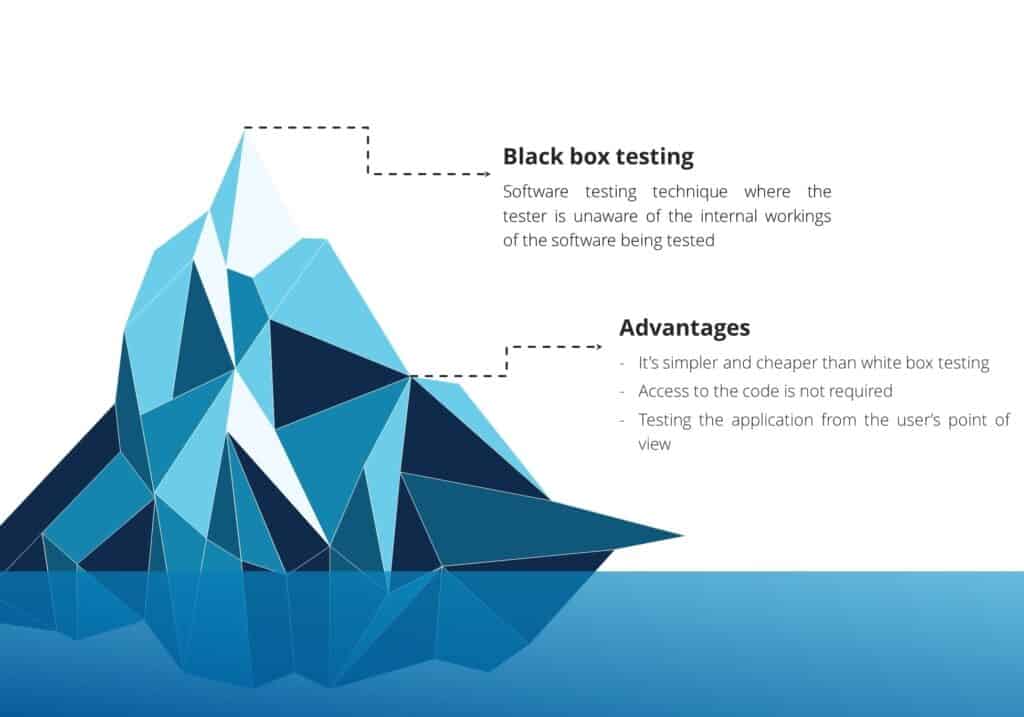
You can use this testing to test a program’s functionality or the usability of a user interface.
Further reading about white and black-box testing techniques.
15. What is gray-box testing?
Gray box testing is a type of software testing that combines black box and white box testing techniques. With gray box testing, testers have partial knowledge of the internal structure of the system under test. This knowledge can come from system documentation or observing the system’s code.
Gray box testing can be used to test individual functions or components of a system. However, you can also use it to test the system as a whole. When used in this way, gray box testing can be used to identify and debug errors in the system.
Gray box testing is a valuable tool for testers and developers. It can help identify errors that may be difficult to find with other testing methods.
Further reading about the gray box testing technique.
16. What is integration testing?
Integration testing is a type of testing used to test the interaction between different software components. It is usually done after unit testing and before system testing. Integration testing ensures that the different software components work together as expected.
There are many different ways to perform integration testing. The most common approach is a bottom-up approach, where the low-level components are tested first, and then the higher-level components are tested. Another common approach is a top-down approach, where the high-level components are tested first, and then the lower-level components are tested.
Integration testing can be used to test both functionality and performance. Functionality testing ensures that the software components work together as expected. Performance testing is used to ensure that the software components perform as expected.
17. What is system testing?
As its name implies, system testing is a type of testing that evaluates a system as a whole. This is in contrast to unit testing, which tests the individual components of a system. System testing is often used to assess the quality of a software product before it is released to customers.
System testing can be performed manually or using automated tools. Testers try to break the system to find bugs or errors when performing system testing. Once all the bugs have been fixed, the system can then be deemed ready for release.
18. What is user acceptance testing?
User acceptance testing (UAT) is a type of testing that is used to ensure that a software application is fit for its purpose. It is usually done by giving the software to a group of people who are representative of the end-users and asking them to use it to see if it meets their needs.
UAT is an integral part of the software development process as it allows developers to get feedback from users on whether the software meets their needs. It also helps to identify any areas where the software needs improvement.
19. What is functional testing?
Functional testing is a type of testing used to assess a system’s functionality. You can use this type of testing to test software, hardware, or any other type of system. Functional testing is typically used to ensure that a system works as intended.
There are many different types of functional testing, but some common methods include unit testing, integration testing, and system testing. Functional testing can be performed manually or automated. Automated functional testing is often used in conjunction with continuous integration and continuous delivery to provide instant feedback on the function of a system.
Functional testing is an important part of ensuring the quality of a system. By testing the functionality of a system, we can identify any bugs or issues that may impact the system’s ability to function correctly.
20. What is non-functional testing?
Non-functional testing is a type of software testing that evaluates the non-functional aspects of a system. These aspects include performance, scalability, security, and more. Non-functional testing is often performed in addition to functional testing, which tests the system’s functionality.
Non-functional testing is important because it ensures the system can handle the load and traffic it will experience in a real-world environment. Without non-functional testing, there is a risk that the system will crash or experience other issues when it is put under real-world conditions.
21. Is unit testing a white box or a black box testing technique?
Unit testing is a white box testing technique. Unit tests have access to the inner workings of the units being tested. This allows for more comprehensive testing, as all unit parts can be tested. However, it also means that unit tests can be more challenging to write, as the developer needs to understand the code being tested.
Further reading about why unit testing is a white box testing technique.
22. What is the best way to unit test a method that returns a void?
The best way to test the void method is to check the side effect after the method has been executed.
You can:
- check the properties that the method has changed
- use mock to verify that the call to the other service has happened
- use an integration test to check the system’s state after executing a void method.
Further reading about how to unit test void method.
23. What is the best way to unit test private methods?
Private methods are implementation details and are subject to change without notice. They are not part of the public API and should not be unit tested. If a private method is needed to implement a public method, it should be fully covered by the public method’s unit tests. There are some rare exceptions to this rule, but private methods should not be unit tested.
Further reading about why not to unit test private methods.
24. What is test-driven development (TDD)?
Test-driven development (TDD) is a process that suggests that software should be developed by writing tests and then writing code to pass those tests. We can summarize the TDD process in three steps:
1. Write a failing test.
2. Implement the functionality to make the test pass.
3. Refactor the code to make it production-ready.

TDD is an iterative process, meaning these steps repeat until the software is complete. The benefit of TDD is that it helps developers focus on the code’s functionality rather than on the code itself.
Further reading about test-driven development.
25. What are the advantages of test-driven development?
There are many advantages to using test-driven development. For one, it helps you catch errors early in the development process. This can save you time and money in the long run, as you won’t have to go back and fix errors that could have been prevented in the first place. In addition, test-driven development can help you create better code, as you will be forced to think about all the different scenarios your code needs to handle.
26. What are the disadvantages of test-driven development?
TDD also has its fair share of disadvantages. For one, writing tests for all the functionality that needs to be developed can be time-consuming. This can lead to frustration among developers, especially if they are under pressure to deliver working software quickly. Additionally, TDD can be difficult to learn and implement, especially for those not used to working in this way. In some cases, it can be problematic to start using it in legacy code.
Despite its drawbacks, TDD is still a popular software development methodology, especially among those who value quality and thoroughness.
27. What is reasonable code coverage for unit testing?
The reasonable code coverage is between 50-80%. Anything below 50% is considered low code coverage and is insufficient to ensure your code is thoroughly tested. On the other hand, anything higher than 80% is considered high code coverage and is likely redundant, as most errors will be found in the 50-80% range.
Further reading about reasonable code coverage.
28. Is unit testing worth the effort?
Unit testing is a vital part of the software development process. It helps to ensure that your code is functioning correctly and that your program meets all the requirements. Unit testing can also save you time and money in the long run by catching errors early on in the development process.
Overall, unit testing is definitely worth the effort. It may take some time to set up, but it will save you time and money in the long run. Plus, knowing that your code is functioning properly will give you peace of mind.
29. What are different code coverage techniques?
There are different types of code coverage, but the most important are:
- function coverage
- statement coverage
- branch coverage
- condition coverage

Further reading about code coverage types.
30. What is refactoring?
Refactoring is the process of making changes to your code without changing its functionality. It can involve anything from restructuring your code to using better variable names.
Many different techniques can be used when refactoring code. Some common ones include Extract Method, Extract Class, Move Method and Replace Nested If Statements With Guard Clause.
31. What is a unit testing framework?
A unit testing framework is a tool that helps developers create and manage unit tests.
When choosing a unit testing framework, you must consider your needs and the programming language you use. Some frameworks are better suited for specific languages or tasks than others.
For example, JUnit is a popular framework for Java applications, while PHPUnit is a popular framework for PHP applications. For .NET, you can use xUnit, NUnit, or MSTest.
32. What is a mocking library?
A mocking library is a tool that helps create fake objects for testing purposes. These objects mimic the behavior of real objects and can be used to test how your code interacts with them. Mocking libraries are instrumental in unit testing, as they can help you isolate the code you are testing and avoid any possible side effects.
There are many different mocking libraries available, each with its own features and benefits. Some of Java’s most popular mocking libraries are Mockito, EasyMock, and PowerMock. For .NET, there are also different options, but one of the most popular is the moq.
33. What is the difference between integration tests and unit tests?
Integration tests are tests that check how different parts of a system work together. Unit tests, on the other hand, are tests that check the functionality of a single component. Both tests are important in ensuring that a system works as intended. However, unit tests are typically used to catch small bugs, while integration tests are used to catch bugs that only occur when different system parts are used together.
Further reading on differences between integration and unit tests
34. What is the difference between functional tests and unit tests?
Functional tests check the overall behavior of a piece of software, while unit tests check the individual units of code that make up the software.
Functional tests are typically high-level tests that check the software’s functionality as a whole. For example, a functional test might check that the software can log in a user, add items to a shopping cart, and checkout.
On the other hand, unit tests are lower-level tests that focus on specific code units. But they can also be written in a way that they test the app on the functional level.
Further reading on differences between unit and functional tests.
35. What is a testing pyramid?
In software testing, there is a concept known as the testing pyramid. The pyramid models how software tests should be structured to achieve efficient and effective testing.
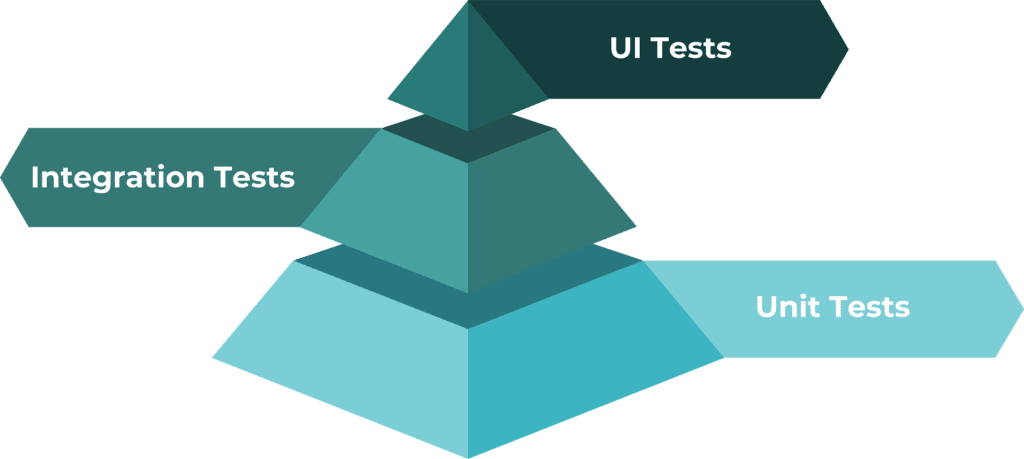
At the base of the pyramid are unit tests. They test the smallest pieces of code (e.g., individual methods or classes). These tests are quick and easy to write and can be run frequently. Next are integration tests, which test how different pieces of code work together. These tests are more expensive and time-consuming to write, but they are still relatively quick and easy to run.
Finally, at the top of the pyramid are UI tests, which test the software’s graphical user interface (GUI). These tests are the most expensive and time-consuming to write, but they are also the most important, as they catch the types of errors that unit and integration tests miss.
Further reading about the testing pyramid.
36. How to unit test static methods?
Static methods are methods that are associated with a class but are not tied to any specific instance of that class. They can be invoked without creating an instance of the class first.
There are three ways to unit test a static method:
- create a wrapper class around it and inject it into class
- use a static Func property
- use the Extract and Override Call refactoring.
Further reading on 3 amazing ways to unit test static method.
37. How many asserts should one unit test contain?
There is no hard and fast rule for how many asserts a unit test should contain. However, as a general guideline, each unit test should focus on testing a single behavior or functionality. Therefore, a unit test should not have more than one assert statement.
Of course, there may be cases where more than one assert statement is necessary. For example, if you are testing a method that returns an object, you will need to assert that each of the properties of that object has the correct value.
Further reading about why multiple asserts are bad.
38. What is the role of unit testing in DevOps?
Unit testing is a crucial part of the DevOps process. It ensures that code changes do not introduce new bugs and that existing functionality is not broken. Unit tests are typically written by the development team and are run automatically as part of the continuous integration and delivery process.

While unit testing is essential, it is just one part of the DevOps puzzle. Other important aspects of DevOps include continuous integration, continuous delivery, and DevOps automation. When all of these pieces work together, they help speed up the software development process and deliver better quality software to customers.
Further reading about the role of unit testing in DevOps.
39. What is a behavior-driven development (BDD)?
Behavior-driven development is an approach to software development that emphasizes the importance of delivering software that meets the customer’s needs. To achieve this, behavior-driven development focuses on creating software based on user stories or descriptions of how the software should work from the end-user’s perspective.
User stories are used to create acceptance criteria, a set of tests that software must meet to be considered complete. These acceptance criteria guide the development process and ensure that the software meets the customer’s needs. Behavior-driven development is an iterative process, which means that new features are added in small increments.
Further reading about BDD and its differences from TDD.
40. What is performance testing?
Performance testing is the process of assessing the response time and stability of a system under load. It is an essential aspect of quality assurance and helps ensure that a system can handle the high volume of traffic it is expected to receive. There are many different types of performance tests, but they all have one goal in common: to identify any potential bottlenecks in a system so they can be addressed before the system goes live.
Load testing, stress testing, and scalability testing are all common types of performance tests. Load testing assesses how a system responds to normal traffic levels. Stress testing assesses how a system responds to peak traffic levels. Finally, scalability testing assesses how well a system can scale up or down to meet changing demands.
41. What is regression testing?
Regression testing is a type of software testing that is used to verify that software changes do not introduce new bugs. This is done by re-running previously completed tests and comparing the results to the expected outcomes. The software change can be successful if the two results match. However, if the results do not match, then the change is considered to have introduced a new bug, and further testing will be needed.
Further reading about differences between regression and unit testing.
42. What is penetration testing?
Penetration testing, also known as pen testing or ethical hacking, is the process of testing a computer system, network, or application to find security vulnerabilities that hackers could exploit. Penetration testing can be used to test internal and external networks and performed by internal or external security teams. The goal of penetration testing is to identify security weaknesses and vulnerabilities so that you can fix them before attackers have a chance to exploit them.
43. What is smoke testing?
Smoke testing is a type of software testing used to identify errors in the software before it is released to the public. This is done by running a series of tests on the software to see if it is stable enough to be used. If the software fails any of the tests, it is deemed unstable and must be fixed before it can be released.
44. What is static testing?
Static testing is a method of testing software that involves inspecting the code without running it. You can do this manually by reading through the code or using automated tools that analyze the code for potential errors.
Static testing can find various errors, including syntax errors, bugs that cause crashes or data loss, and security vulnerabilities. You can also use it to check for compliance with coding standards.
45. What is dynamic testing?
Dynamic testing is a method of testing software that involves executing the software in an actual or simulated environment and observing its behavior. Developers or testers usually perform this type of testing during the development process.
Dynamic testing can test the functionality of the software, as well as its performance and stability. In addition, this type of testing is often used to find bugs or defects in the software. Dynamic testing can be used to test both unit-level and system-level code.
46. What is API testing?
API testing is a type of software testing that focuses on the application programming interfaces (APIs) that are used to communicate between different software systems. API testing can test applications that use APIs to communicate with each other and applications that expose APIs to be used by third-party developers.

Further reading on differences between API and unit testing.
47. What is alpha testing?
Alpha testing is a type of software testing carried out at a software product’s development stage. It is the first testing stage and is usually done by the development team before the software is released to beta testers.
The purpose of alpha testing is to find any major bugs or issues with the software before it is released to the general public. Doing this helps ensure that the software is of high quality and is more likely to succeed when it is finally released.
48. What is beta testing?
Beta testing is the process of testing a product or service before it is released to the public. You can do this with a small group of users or on a larger scale with a limited release. Beta testing is a helpful way to gather feedback from potential users and ensure that the product or service is ready for launch.
49. What is acceptance test-driven development?
Acceptance test-driven development (ATDD) is a variation of TDD that focuses on writing tests that describe the system’s behavior from the user’s perspective. They ensure that the system meets the needs of the users and that it is easy to use.
Further reading about acceptance test-driven development.
50. How to unit test legacy code?
One of the challenges of unit testing is dealing with legacy code. It is code that was written without tests and is not testable. However, there are ways you can overcome this challenge and unit test legacy code.
There is no one right way to unit test legacy code. It depends on the code itself and the team working on it. However, with some refactoring knowledge and perseverance, it is possible to get legacy code under test.
Some actionable steps are:
- Write characterization tests first.
- Run characterization tests in a CI/CD process.
- Start with easy-to-test legacy code if the team has no previous knowledge about refactoring and unit testing. Start with hard-to-test legacy code if the team has experience with refactoring and unit testing.
Further reading about the legacy code and action plan to write tests for it.
Conclusion
These 50 unit testing interview questions cover a wide range of topics. They are designed to test your knowledge of unit testing concepts and your ability to apply them in a real-world setting. With these questions, you should be well-prepared for your next interview.
If you need a list of 100 C# interview questions, here it is.
Good luck!